8.1 结构体基本概念
结构体属于用户自定义的数据类型,允许用户存储不同的数据类型。
8.2 结构体定义和使用
1、定义结构体的语法:struct 结构体名 {结构体成员列表};
例子:
struct student
{
string name;
int age;
float score;
};
2、创建结构体变量的方式 创建结构体变量的方式有三种:(推荐第一种和第二种)
- struct 结构体名 变量名;
- struct 结构体名 变量名={成员1值, 成员2值,…};
- 定义结构体时顺便创建变量。
(1)struct 结构体名 变量名; 例子:
struct student s1;
s1.name = "张三";
s1.age = 18;
s1.score = 100;
(2)struct 结构体名 变量名={成员1值, 成员2值,…}; 例子:
struct student s2 = { "李四", 19,80 };
(3)定义结构体时顺便创建变量 例子:
struct student
{
string name;
int age;
float score;
}s3;
3、使用结构体变量 通过在结构体变量后面加上
?
\cdot
? 来访问结构体变量中的属性。 例子:
s1.name = "张三";
s1.age = 18;
s1.score = 100;
cout << "姓名:" << s1.name
<< " 年龄:" << s1.age
<< " 分数:" << s1.score << endl;
注意:在输出sring类型的数据时,在头文件处要包含#include< string >
示例:
#include<iostream>
#include<string>
using namespace std;
struct student
{
string name;
int age;
float score;
}s3;
int main()
{
struct student s1;
s1.name = "张三";
s1.age = 18;
s1.score = 100;
cout << "姓名:" << s1.name << " 年龄:" << s1.age << " 分数:" << s1.score << endl;
struct student s2 = { "李四", 19,80 };
cout << "姓名:" << s2.name << " 年龄:" << s2.age << " 分数:" << s2.score << endl;
s3.name = "王五";
s3.age = 20;
s3.score = 60;
cout << "姓名:" << s3.name << " 年龄:" << s3.age << " 分数:" << s3.score << endl;
system("pause");
return 0;
}
运行结果: 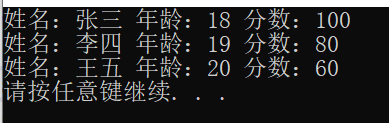 4、总结: (1)定义结构体时,关键字struct不可以省略;创建结构体变量时,关键字struct可以省略。 (2)结构体变量用操作符 " . " 访问成员。
8.3 结构体数组
1、作用:将自定义的结构体放入到数组中方便维护。 2、语法:struct 结构体名 数组名 [元素个数]={ { } , { } ,… { } }; 例子: 在定义好“student”这个结构体后 (1)创建结构体数组
struct student stuArry[3]=
{
{"张三",18,100},
{"李四",28,99},
{"王五",38,66}
};
(2)给结构体中的元素赋值
stuArry[2].name = "赵六";
stuArry[2].age = 80;
stuArry[2].score = 60;
(3)遍历结构体
for (int i = 0; i < 3; i++)
{
cout << "姓名:" << stuArry[i].name
<< " 年龄:" << stuArry[i].age
<< " 分数:" << stuArry[i].score << endl;
}
示例:
#include<iostream>
#include<string>
using namespace std;
struct student
{
string name;
int age;
float score;
};
int main()
{
struct student stuArry[3]=
{
{"张三",18,100},
{"李四",28,99},
{"王五",38,66}
};
stuArry[2].name = "赵六";
stuArry[2].age = 80;
stuArry[2].score = 60;
for (int i = 0; i < 3; i++)
{
cout << "姓名:" << stuArry[i].name
<< " 年龄:" << stuArry[i].age
<< " 分数:" << stuArry[i].score << endl;
}
system("pause");
return 0;
}
运行结果: 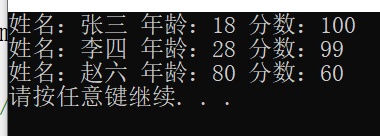
8.4 结构体指针
1、作用:通过指针访问结构体中的成员。 利用操作符 “ -> ” ,可以通过结构体指针访问结构体属性。 例子:
在定义了 “ student "这个结构体和创建了结构体变量struct student s = { “张三”,18,100 },后,定义结构体指针:
struct student * p = &s;
示例:
#include<iostream>
#include<string>
using namespace std;
struct student
{
string name;
int age;
float score;
};
int main()
{
struct student s = { "张三",18,100 };
struct student * p = &s;
cout << "姓名:" << s.name << " 年龄:" << s.age << " 分数:" << s.score << endl;
cout << "姓名:" << p->name << " 年龄:" << p->age << " 分数:" << p->score << endl;
system("pause");
return 0;
}
运行结果: 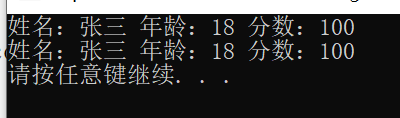
8.5 结构体嵌套结构体
作用:结构体的成员可以是另一个结构体。 例如:每个老师辅导一个学员,一个老师的结构体中,记录了一个学生的结构体。 例子:
1、定义学生结构体
struct student
{
string name;
int age;
float score;
};
2、定义老师结构体
struct teacher
{
int id;
string name;
int age;
struct student stu;
};
3、在主函数之中创建老师
struct teacher t;
t.id = 10000;
t.name = "老王";
t.age = 50;
t.stu.name = "小王";
t.stu.age = 20;
t.stu.score = 60;
完整示例:
#include<iostream>
#include<string>
using namespace std;
struct student
{
string name;
int age;
float score;
};
struct teacher
{
int id;
string name;
int age;
struct student stu;
};
int main()
{
struct teacher t;
t.id = 10000;
t.name = "老王";
t.age = 50;
t.stu.name = "小王";
t.stu.age = 20;
t.stu.score = 60;
cout << "老师的姓名:" << t.name
<< " 老师的编号:" << t.id
<< " 老师的年龄:" << t.age
<< endl;
cout<< "老师辅导的学生姓名: " << t.stu.name
<< " 老师辅导学生的年龄: " << t.stu.age
<< " 老师辅导学生的分数: " << t.stu.score
<< endl;
system("pause");
return 0;
}
运行结果: 
8.6 结构体做函数参数
作用:将结构体作为参数向函数中传递。 传递方式有两种:
前提条件: 定义了“student”的这一结构体,并且在主函数中,定义了一个 student类型的变量,变量名为stu,而且还进行了赋值。 (1)定义“student”的这一结构体
struct student
{
string name;
int age;
float score;
};
(2)在main()函数中,定义并赋值了一个 student类型的结构体变量
struct student s;
s.name = "张三";
s.age = 20;
s.score = 80;
要创建打印学生信息的函数
8.6.1 值传递
void printstudent1(struct student s)
{
s.age = 100;
cout << "在子函数1中打印 姓名:" << s.name << " 年龄:" << s.age << " 考试分数: " << s.score << endl;
}
在main()输入定义好的结构体变量:
int main()
{
struct student s;
s.name = "张三";
s.age = 20;
s.score = 80;
printstudent1(s);
cout << "在main()函数中打印 姓名:" << s.name
<< " 年龄:" << s.age
<< " 考试分数: " << s.score
<< endl;
system("pause");
return 0;
}
运行结果:  可以看到,值传递只是在子函数中改变了形参的值,将年龄变为100,而实参的值并没有发生改变,依旧是20。
8.6.2 地址传递
void printstudent2(struct student *p)
{
p->age = 100;
cout << "在子函数2中打印 姓名:" << p->name << " 年龄:" <<p->age << " 考试分数: " <<p->score << endl;
}
在main()函数中输入定义好的结构体变量的地址:
int main()
{
struct student s;
s.name = "张三";
s.age = 20;
s.score = 80;
printstudent2(&s);
cout << "在main()函数中打印 姓名:" << s.name
<< " 年龄:" << s.age
<< " 考试分数: " << s.score
<< endl;
system("pause");
return 0;
}
运行结果:  可以看到,地址传递不仅在子函数中改变了形参的值,将年龄从20变为100,也将主函数中实参的值也进行了改变。
完整示例:
#include<iostream>
#include<string>
using namespace std;
struct student
{
string name;
int age;
float score;
};
void printstudent1(struct student s)
{
s.age = 100;
cout << "在子函数1中打印 姓名:" << s.name << " 年龄:" << s.age << " 考试分数: " << s.score << endl;
}
void printstudent2(struct student *p)
{
p->age = 100;
cout << "在子函数2中打印 姓名:" << p->name << " 年龄:" <<p->age << " 考试分数: " <<p->score << endl;
}
int main()
{
struct student s;
s.name = "张三";
s.age = 20;
s.score = 80;
printstudent2(&s);
cout << "在main()函数中打印 姓名:" << s.name << " 年龄:" << s.age << " 考试分数: " << s.score << endl;
system("pause");
return 0;
}
总结:如果不想修改主函数当中的数据,用值传递,反之则用地址传递。
8.7 结构体中const的使用场景
作用:用const防止误操作。
前提:定义好了”student"的结构体
定义了一个常量指针,如下:
void printstudent(const struct student *s)
{
}
 可以看到,在定义了常量指针后,不能对参数进行修改,否则报错。
完整示例:
#include<iostream>
#include<string>
using namespace std;
struct student
{
string name;
int age;
float score;
};
void printstudent(const struct student *s)
{
cout << "姓名:" << s->name << " 年龄:" << s->age << " 分数:" << s->score << endl;
}
int main()
{
struct student s = { "张三",15,70 };
printstudent(&s);
system("pause");
return 0;
}
|