1.编写程序,根据公式
π
4
=
1
?
1
3
+
1
5
?
1
7
+
1
7
.
.
.
.
.
\frac{\pi}{4} = 1-\frac{1}{3}+\frac{1}{5}-\frac{1}{7}+\frac{1}{7}.....
4π?=1?31?+51??71?+71?.....计算圆周率
π
\pi
π的值,直到公式最后一项的绝对值小于
1
0
?
6
10^{-6}
10?6。
#include <iostream>
using namespace std;
#include <cmath>
int main(){
const double eps= 1e-6;
double pi=0.0;
int sign = 1;
double item = 1;
double d = 1.0;
while (fabs(item) >= eps)
{
pi += item;
sign = -sign;
d += 2.0;
item = sign * (1 / d);
}
pi *= 4;
cout << "PI 的值为:" << pi << endl;
return 0;
}
结果输出为:
PI 的值为:3.14159
2.使用循环结构编写程序,显示如下由字符“*”组成的图形 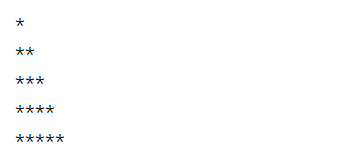
#include <iostream>
using namespace std;
#include <cmath>
int main(){
for (int i = 0; i <= 5; i++)
{
for (int j = 0; j <= i; j++)
cout << '*';
cout << '\n';
}
return 0;
}
3.编写一个统计学生平均分数的程序,要求:首先输入一名学生的五门课程分数,计算其平均分数并输出,然后提示用户是否退出程序,键入’Y’或’y’程序会退出,键入其他字母程序将继续统计下一名学生的平均分数。
#include <iostream>
using namespace std;
#include <cmath>
int main(){
const int NUM = 5;
int count= 0;
bool quit = false;
do {
double sum = 0, g;
cout << "Input 5 grades:" << endl;
for (int i = 0; i < NUM; i++) {
cin >> g;
sum += g;
}
count++;
double avg = sum / NUM;
cout << "No." << count << "AVG is " << avg << endl;
char ch;
cout << "Want to quit(YES='Y'or 'y')?";
cin>>ch;
if((ch=='Y') || (ch = 'y')) {
quit = true;
}
} while (!quit);
return 0;
}
4.打出九九乘法表
#include <iostream>
using namespace std;
#include <cmath>
for (int i = 1; i < 10; i++) {
for (int j = 1; j < 10; j++) {
if (j == i + 1)
break;
cout << i * j << '\t';
}
cout << '\n';
}
return 0;
}
输出结果为:
1
2 4
3 6 9
4 8 12 16
5 10 15 20 25
6 12 18 24 30 36
7 14 21 28 35 42 49
8 16 24 32 40 48 56 64
9 18 27 36 45 54 63 72 81
5.编写程序,实现输入一个整数,判断其能否被3,5,7整除,并输出一下信息之一。 (a)能同时被3,5,7整除 (b)能被其中两个数整除(要输出是哪两个)整除 (c)不能被3,5,7中的任一个数整除
#include <iostream>
using namespace std;
int main() {
int a;
int b = 3, c = 5, d = 7;
cout << "Please input an integer:" << endl;
cin >> a;
if((a%b==0)&&(a%c==0)&&(a%d==0))
{
cout << "能同时被3,5,7整除" << endl;
}
else if ((a % b != 0) && (a % c == 0) && (a % d == 0))
{
cout<<"能被其中两个数"<<c<<d<<"整除"<<endl;
}
else if ((a % b == 0) && (a % c != 0) && (a % d == 0))
{
cout << "能被其中两个数" << b << d << "整除" << endl;
}
else if ((a % b == 0) && (a % c == 0) && (a % d != 0))
{
cout << "能被其中两个数" << b << c << "整除" << endl;
}
else {
cout << "不能被" << b << c << d << "中的任意一个数整除" << endl;
}
return 0;
}
6.将一个Fibonacci序列赋值给一个数组。Fibonacci数列:
a
n
=
a
n
?
1
+
a
n
?
2
a_{n} =a_{n-1}+a_{n-2}
an?=an?1?+an?2?。
#include <iostream>
using namespace std;
#include <cmath>
int main() {
int Fibo[20];
Fibo[0] = 1;
Fibo[1] = 1;
for (int i = 2; i < 20; i++) {
Fibo[i] = Fibo[i - 1] + Fibo[i - 2];
}
cout << "Fibonacci数列为:" << endl;
for (int j = 0; j < 20; j++) {
cout << Fibo[j] <<' ';
}
return 0;
}
7.创建一个含有五个元素的数组,并给每个数组元素赋初值。接着计算这些值的平均数,并找出最大值和最小值。
#include <iostream>
using namespace std;
int main() {
const int arraysize = 5;
int g[arraysize] = { 1,8,56,94,3, };
int sum = 0;
int ave;
int min, max;
for (int i = 0; i < 5; i++) {
sum += g[i];
}
ave = sum / arraysize;
min=max = g[0];
for (int j = 0; j < 5; j++) {
if (g[j] > max)
max = g[j];
if (g[j] < min)
min = g[j];
}
cout << "这些值的平均值为:" << ave << endl;
cout << "最大值为:" << max << endl;
cout << "最小值为:" << min << endl;
return 0;
}
8.演示new和delete的用法
#include <iostream>
#include <cstdlib>
using namespace std;
int main() {
int arraySize;
int *array;
cout << "Please input the size of the array:";
cin >> arraySize;
array = new int[arraySize];
if (arraySize == NULL) {
cout << "Cannot allocate more memory, exit the program.\n";
exit(1);
}
for (int i = 0; i < arraySize; i++) array[i] = i * i;
for (int i = 0; i < arraySize; i++) cout << array[i] << " ";
cout << endl;
delete[]array;
return 0;
}
输出结果为
Please input the size of the array:11
0 1 4 9 16 25 36 49 64 81 100
9.阶乘函数的实现。 例一:
#include <iostream>
#include <cstdlib>
using namespace std;
int fact(int n);
int main() {
int a;
cout <<"Please input a number:" << endl;
cin >> a;
cout << fact(a) << endl;
return 0;
}
int fact(int n) {
int f = 1;
while (n) {
f *= n;
n--;
}
return f;
}
例二:(递归函数的实现)
#include <iostream>
#include <cstdlib>
using namespace std;
int fact(int n)
{
if (n <= 1) return 1;
else return n * fact(n - 1);
}
int main() {
int k;
do {
cout << "请输入一个非负数:" << endl;
cin >> k;
} while (k < 0);
cout << "K的阶乘为:" << fact(k) << endl;
}
10.汉诺塔问题:
#include <iostream>
using namespace std;
void hanoi(int n, char x, char y, char z);
int main()
{
int n;
cout << "Please input the number of hanoi tower:" << endl;
cin >> n;
hanoi(n, 'A', 'B', 'C');
return 0;
}
void hanoi(int n, char x, char y, char z)
{
if (n > 0)
{
hanoi(n - 1, x, y, z);
cout << "MOVE" << n << ": " << x << "->>" << z << endl;
hanoi(n - 1, y, x, z);
}
}
当盘子数为3时,输出结果为:
Please input the number of hanoi tower:
3
MOVE1: A->>C
MOVE2: A->>C
MOVE1: B->>C
MOVE3: A->>C
MOVE1: B->>C
MOVE2: B->>C
MOVE1: A->>C
11.在类中定义并使用静态数据成员的实例
#include <iostream>
using namespace std;
class Point
{
public:
Point(int xx = 0, int yy = 0, int zz = 0) :X(xx), Y(yy), Z(zz)
{
count++;
}
void display()
{
cout << "the point is:(" << X << ',' << Y << ',' << Z << ')'<<endl;
cout << "the count is:" << count << endl;
}
private:
int X, Y, Z;
static int count;
};
int Point::count = 0;
int main()
{
Point p1(4, 8, 9), p2(99, 56, 45);
p1.display();
p2.display();
return 0;
}
输出结果为:
the point is:(4,8,9)
the count is:2
the point is:(99,56,45)
the count is:2
12.普通函数作为类的友元函数
#include <iostream>
using namespace std;
class TIME;
class DATE
{
public:
DATE( int xx, int yy, int zz) :Year(xx), Month(yy), Day(zz){}
friend void DATETIME(const DATE &d, const TIME &t);
private:
int Year, Month, Day;
};
class TIME
{
public:
TIME(int h, int m, int s) :Hour(h), Minute(m), Second(s) {}
friend void DATETIME(const DATE &d, const TIME &t);
private:
int Hour, Minute, Second;
};
void DATETIME(const DATE &d, const TIME &t)//友元函数为普通函数,是类外定义的
{
cout << "Now is :" << d.Year << '.'<<d.Month << '.'<<d.Day << '.'<<t.Hour << '.'<<t.Minute << '.'<<t.Second << endl;
}
int main()
{
DATE d(2021, 8, 5);
TIME t(21, 6, 45);
DATETIME(d, t);
return 0;
}
13.派生类析构函数的执行过程如下示例.
#include<iostream>
using namespace std;
class Base
{
public:
Base() :b1(0), b2(0) {}
Base(int i, int j);
~Base();
void Print()const { cout << b1 << "," << b2 << "," << endl; }
private:
int b1, b2;
};
Base::Base(int i, int j) :b1(i), b2(j)
{
cout << "Base's constructor called." << b1 << "," << b2 << endl;
}
Base::~Base()
{
cout << "Base's destructor called." << b1 << "," << endl;
}
class Derived :public Base
{
public:
Derived() :d(0) {}
Derived(int i, int j, int k);
~Derived();
void Print() const;
private:
int d;
};
Derived::Derived(int i, int j, int k) :Base(i, j), d(k)
{
cout << "Derived;s constructor called." << d << endl;
}
Derived::~Derived()
{
cout << "Derived's destructor called." << d << endl;
}
void Derived::Print()const
{
Base::Print();
cout << d << endl;
}
int main()
{
Derived objD1(1, 2, 3), objD2(-4, -5, -6);
objD1.Print();
objD2.Print();
return 0;
}
14.实现一个小的图形系统,它只能处理两种图形:Rectangle和Circle。每一种图形都涉及两个基本操作:求出图形的面积和画出图形,这两个操作分别命名为area和draw.
|