普通构造函数类
- CMatrix()
没有参数的函数构造,函数源码为:
CMatrix::CMatrix() :m_nRow(0), m_nCol(0), m_pData(0){
}
另一种构造方式为:
CMstrix::CMatrix(){
m_nRow=0;
m_nCol=0;
m_pData=0;
}
- CMatrix(int nRow, int nCol, double* pData = NULL)
有参数的构造函数,构造时可以知道行数、列数以及矩阵内的具体值 注:m_pData(0)与m_pData= NULL等价
CMatrix::CMatrix(int nRow, int nCol, double* pData) : m_pData(0) {
Create(nRow, nCol, pData);
}
- CMatrix(const CMatrix& m);
构造一个函数,将括号内的矩阵拷贝至括号外 有三种不同的构造方法
CMatrix::CMatrix(const CMatrix& m) : m_pData(0) {
*this = m;
}
CMatrix::CMatrix(const CMatrix& m) {
m_nRow = m.m_nRow;
m_nCol = m.m_nCol;
m_pData = m.NULL;
Create(m_nRow,m_nCol,m.m_npData);
}
CMatrix::CMatrix(const CMatrix& m) : CMatrix(m.m_nRow,m.m_nCil,m_npData) {
}
- CMatrix(const char * strPath):文件流读取函数
- 注:this:成员函数中都有this指针,指向本身对象的地址
this指针加变成对象 this指针加&变成地址
CMatrix::CMatrix(const char* strPath) {
m_pData = 0;
m_nRow = m_nCol = 0;
ifstream cin(strPath);
cin >> *this;
}
- ~CMatrix():析构函数
主要用于删除内存时使用,既当某一函数为虚构函数时,删除其内存 release()函数将在下文提到,本质是删除内存语句
CMatrix::~CMatrix() {
Release();
}
其他的构造函数
- 创建函数:bool Create(int nRow, int nCol, double* Data = NULL)
bool CMatrix::Create(int nRow, int nCol, double* pData) {
Release();
m_pData = new double[nRow * nCol];
m_nRow = nRow;
m_nCol = nCol;
if (pData) {
return memcpy(m_pData, pData, nRow * nCol * sizeof(double));
}
}
- 修改矩阵值:void Set(int nRow, int nCol, double dVale)
此函数的作用为指定修改将第几行第几列的值修改为赋予的值 因为函数内部语句很少所以使用内联函数提高效率 内联函数的规格应该不超过十行,并且递归、循环函数不能作为内联函数
inline void CMatrix::Set(int nRow, int nCol, double dVal) {
m_pData[nRow * m_nCol + nCol] = dVal;
}
void CMatrix::Set(int nRow, int nCol, double dVal) {
m_pData[nRow * m_nCol + nCol] = dVal;
}
- 释放内存:void Release()
void CMatrix::Release() {
if (m_pData) {
delete[]m_pData;
m_pData = NULL;
}
m_nRow = m_nCol = 0;
}
输入输出流的重构
加上friend后两个函数可以放在内部,依然是全局函数而不是成员函数,只是赋予了改函数访问所有变量的特权
friend istream & operator>>(istream& is, CMatrix& m);
friend ostream& operator<<(ostream& os, const CMatrix& m);
- 输出:istream & operator>>(istream& is, CMatrix& m)
istream& operator>>(istream& is, CMatrix& m) {
is >> m.m_nRow >> m.m_nCol;
m.Create(m.m_nRow, m.m_nCol);
for (int i = 0; i < m.m_nRow * m.m_nCol; i++) {
is >> m.m_pData[i];
}
return is;
}
- 输出:ostream& operator<<(ostream& os, const CMatrix& m)
ostream& operator<<(ostream& os, const CMatrix& m) {
os << m.m_nRow << "行" << m.m_nCol <<"列"<< endl;
double* pData = m.m_pData;
for (int i = 0; i < m.m_nRow; i++) {
for (int j = 0; j < m.m_nCol; j++) {
os << *pData++ << "";
}
os << endl;
}
return os;
}
运算符重构
- 赋值:CMatrix& operator = (const CMatrix& m)
CMatrix& CMatrix::operator=(const CMatrix& m) {
if (this != &m) {
Create(m.m_nRow, m.m_nCol, m.m_pData);
}
return *this;
}
- +=:CMatrix& operator += (const CMatrix& m)
CMatrix& CMatrix::operator+=(const CMatrix& m) {
assert(m_nRow == m.m_nRow && m_nCol == m.m_nCol);
for (int i = 0; i < m_nRow * m_nCol; i++) {
m_pData[i] += m.m_pData[i];
}
return *this;
}
- 判断是否等于:bool operator == (const CMatrix& m)
bool CMatrix::operator==(const CMatrix& m) {
if (!(m_nRow == m.m_nRow && m_nCol == m.m_nCol)) {
return false;
}
for (int i = 0; i < m_nRow * m_nCol; i++) {
if (m_pData[i] != m.m_pData[i]) {
return false;
}
}
return true;
}
- 不等于: bool operator != (const CMatrix& m)
bool CMatrix::operator!=(const CMatrix& m) {
return !((*this) == m);
}
- 下标:double& operator[](int nIndex)
double& CMatrix::operator[](int nIndex) {
assert(nIndex < m_nRow * m_nCol);
return m_pData[nIndex];
}
- 圆括号:double& operator()(int nRow, int nCol)
可以输入无数的值
double& CMatrix::operator()(int nRow,int nCol) {
assert(nRow*m_nCol+nCol<m_nRow*m_nCol);
return m_pData[nRow*m_nCol+nCol];
}
- 加号:CMatrix operator+(const CMatrix& m1, const CMatrix& m2);
此函数为全局函数
CMatrix operator+(const CMatrix& m1, const CMatrix& m2) {
CMatrix m3(m1);
m3 += m2;
return m3;
}
强制转换函数
operator double()
CMatrix::operator double() {
double ds = 0;
for (int i = 0; i < m_nRow * m_nCol; i++) {
ds += m_pData[i];
}
return ds;
}
主函数源码
#include <iostream>
#include "CComplex.h"
#include<stdio.h>
#include"CMatrix.h"
using namespace std;
int main(int argc, char** argv) {
CMatrix m1;
cout << "无参构造m1 :" << m1 << endl;
double pData[10] = { 2,3,4,5 };
CMatrix m2(2, 5, pData);
cout <<"有参构造m2: "<< m2 << endl;
CMatrix m3("d://1.txt");
cout << "读取文件流m3: " << m3 << endl;
CMatrix m4(m2);
cout << "拷贝复制m4 :" << m4 << endl;
m2.Set(0, 2, 10);
cout << "修改后m2 :" << m4 << endl;
m1 = m2;
cout << "修改后m1 :" << m4 << endl;
if (m4 == m2) {
cout << "Erroe!" << endl;
}
m4 += m1;
cout << "sum of m4 = " << m4 << endl;
cout << "强制转换 m4=" << (double)m4 << endl;
return 0;
}
所有函数的运行结果
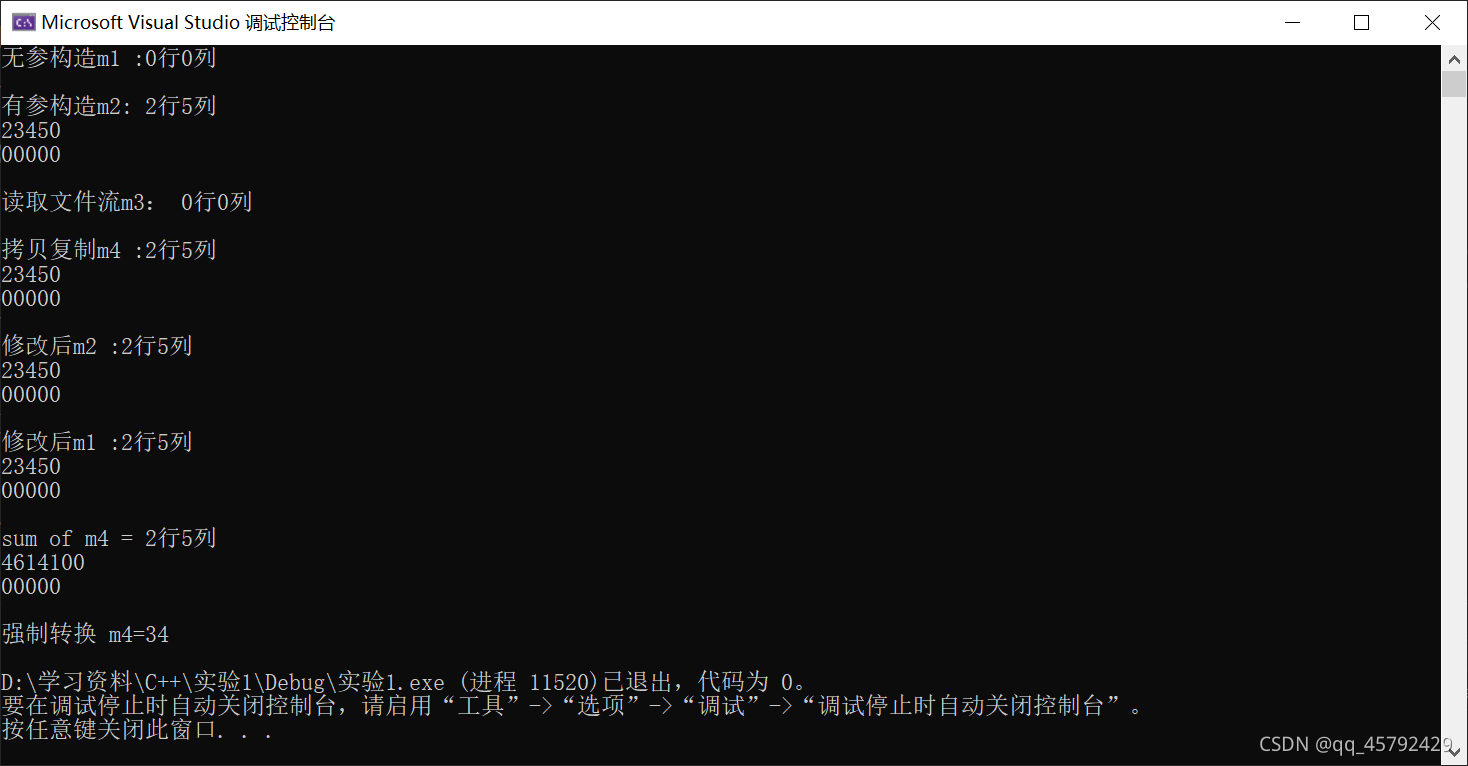
|