目录
map 基本概念
map 构造和赋值
map 容器大小和交换
map 插入和删除
map 查找和统计
map 排序
map 基本概念
简介:
- map 中所有元素都是 pair
- pair 中第一个元素为 key (键值),起到索引作用,第二个元素为 value (实值)
- 所有元素都会根据元素的键值自动排序
- 需要头文件 <map>
本质:
- map / multimap 属于关联式容器,底层结构是二叉树实现
优点:
map 和?multimap 区别:
- map 不允许容器中有重复 key 值元素
- multimap 允许容器中有重复 key 值元素
map 构造和赋值
功能:
函数原型:
构造
// map 默认构造函数
map<T1, T2> mp;
// 拷贝构造函数
map(const map& mp);
赋值
// 重载 = 操作符
map& operator=(const map& mp);
测试代码:
#include<iostream>
using namespace std;
#include<map>
void PrintMap(map<int,int>& m) {
for (map<int, int>::iterator it = m.begin(); it != m.end(); ++it) {
// (*it).first it->first
cout << "key:" << (*it).first << "value:" << it->second << endl;
}
cout << endl;
}
void test() {
// 创建 map 容器,模板参数有两个
map<int, int>m;
m.insert(pair<int, int>(1, 10)); // 匿名对组,key 为 1,value 是 10
m.insert(pair<int, int>(2, 20));
m.insert(pair<int, int>(4, 40));
m.insert(pair<int, int>(3, 30));
// 会按照 key 自动排序
PrintMap(m);
// 拷贝构造
map<int, int>m2(m);
PrintMap(m2);
// 重载 = 操作符
map<int, int>m3;
m3 = m2;
PrintMap(m3);
}
int main() {
test();
system("pause");
return 0;
}
运行结果:
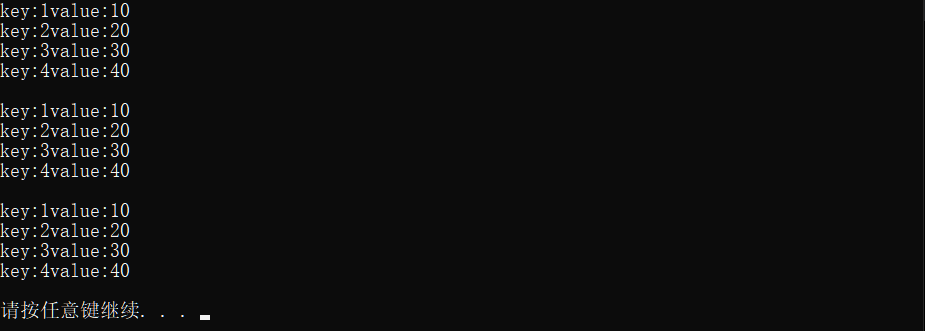
map 中所有元素都是成对出现的,插入数据时要使用对组?
map 容器大小和交换
功能:
函数原型:
// 返回容器中元素的数目
size();
// 判断容器是否为空
empty();
// 交换两个集合容器
swap(st);
测试代码:
#include<iostream>
using namespace std;
#include<map>
size_t Map_size(const map<int, int>& m);
// 打印 map 容器元素
void PrintMap(map<int,int>& m) {
for (map<int, int>::iterator it = m.begin(); it != m.end(); ++it) {
// (*it).first it->first
cout << "key:" << (*it).first << "value:" << it->second << endl;
}
cout << endl;
}
// 判断 map 容器是否为空
void Map_empty(const map<int, int>& m) {
// 判断容器是否为空
if (m.empty()) {
cout << "m 容器为空" << endl;
}
else {
cout << "m 容器非空" << endl;
cout << "map 容器中元素个数:" << Map_size(m) << endl;
}
}
size_t Map_size(const map<int, int>& m) {
return m.size();
}
void test() {
map<int, int>m1;
Map_empty(m1); // 为空
// 插入一个数据
m1.insert(pair<int, int>(1, 10));
Map_empty(m1); // 非空
cout << endl << "m1打印" << endl;
PrintMap(m1);
// 交换
map<int, int>m2;
m2.insert(pair<int, int>(1, 10));
m2.insert(pair<int, int>(2, 20));
m2.insert(pair<int, int>(3, 30));
m2.insert(pair<int, int>(4, 40));
m2.insert(pair<int, int>(5, 50));
cout << "m2交换前:" << endl;
PrintMap(m2);
cout << "m2交换后:" << endl;
m2.swap(m1);
PrintMap(m2);
}
int main() {
test();
system("pause");
return 0;
}
运行结果:
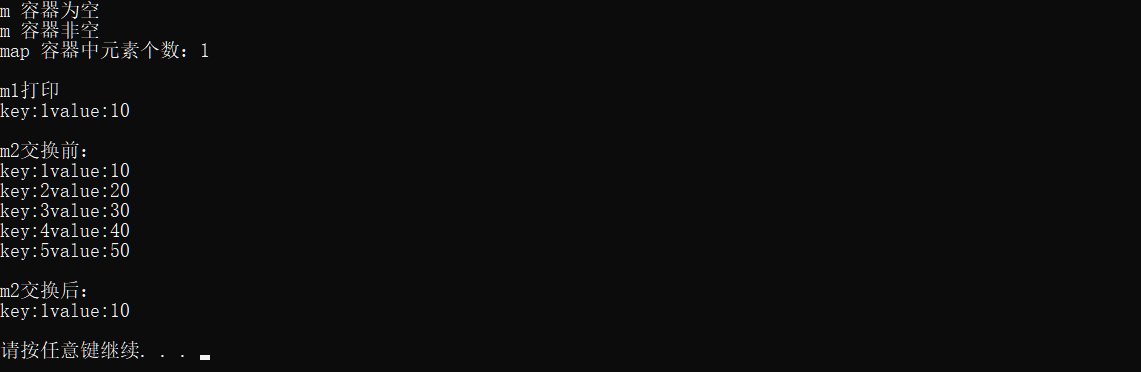
map 插入和删除
功能:
函数原型:
// 在容器中插入元素
insert(elem);
// 清除所有元素
clear();
// 删除 pos 迭代器所指的元素,返回下一个元素的迭代器
erase(pos);
// 删除区间 [beg, end) 的所有元素,返回下一个元素的迭代器
erase(beg, end);
// 删除容器中值为 key 的元素
erase(key);
测试代码:
#include<iostream>
using namespace std;
#include<map>
void PrintMap(map<int,int>& m) {
for (map<int, int>::iterator it = m.begin(); it != m.end(); ++it) {
cout << "key:" << (*it).first << "value:" << it->second << endl;
}
cout << endl;
}
void test() {
map<int, int>m1;
// 插入
// 第一种
m1.insert(pair<int, int>(1, 10));
// 第二种
m1.insert(make_pair(2, 20));
// 第三种
m1.insert(map<int, int>::value_type(3, 30));
// 第四种
m1[4] = 40; // 不建议用来插入,可以用来访问(但要确定存在)
// key = 5 value = 0
//cout << m1[5] << endl; // 因为 key = 5 不存在,所以他会自己创建数据
PrintMap(m1);
// 删除 erase(beg); 传入迭代器
m1.erase(m1.begin());
PrintMap(m1); // key = 10 被删除
// earse(key); 传入 key,把 key 对应的值删除
m1.erase(4);
PrintMap(m1);
// erase(beg, end);
m1.erase(m1.begin(), m1.end()); // 等于清空,方向迭代器
PrintMap(m1);
// 清空
m1.clear();
PrintMap(m1);
}
int main() {
test();
system("pause");
return 0;
}
?运行结果:
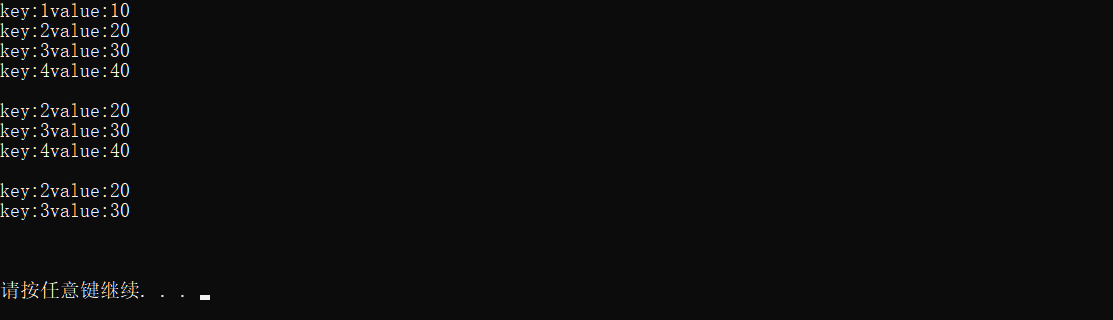
map 查找和统计
功能:
函数原型:
// 查找 key 是否存在
// 存在,返回该键的元素的迭代器
// 不存在,返回 map.end();
find(key);
// 统计 key 的元素个数
count(key);
测试代码:
#include<iostream>
using namespace std;
#include<map>
void test() {
map<int, int>m1;
m1.insert(make_pair(1, 10));
m1.insert(make_pair(2, 20));
m1.insert(make_pair(3, 30));
m1.insert(make_pair(4, 40));
// 查找
map<int, int>::iterator pos = m1.find(3);
if (pos != m1.end()) {
cout << "找到 " << "key = " << pos->first << " value = " << (*pos).second << endl;
}
else {
cout << "未找到" << endl;
}
// 统计,map 容器不允许有相同的 key
// map 只能是 0 或 1
// multimap count 可以 > 1
int num = m1.count(1);
cout << "num = " << num << endl; // 1
}
int main() {
test();
system("pause");
return 0;
}
运行结果:

map 排序
map 容器默认排序规则为按照 key 从小到大排序,如何改变排序规则?
测试代码:
#include<iostream>
using namespace std;
#include<map>
class MyCompare {
public:
bool operator()(int value1, int value2)const {
return (value1 > value2);
}
};
void test() {
map<int, int>m1;
m1.insert(make_pair(5, 50));
m1.insert(make_pair(2, 20));
m1.insert(make_pair(4, 40));
m1.insert(make_pair(3, 30));
m1.insert(make_pair(1, 10));
// 默认升序
for (map<int, int>::iterator it1 = m1.begin(); it1 != m1.end(); ++it1) {
cout << "key = " << (*it1).first << " value = " << it1->second << endl;
}
cout << endl;
// 降序
map<int, int, MyCompare>m2;
m2.insert(make_pair(5, 50));
m2.insert(make_pair(2, 20));
m2.insert(make_pair(4, 40));
m2.insert(make_pair(3, 30));
m2.insert(make_pair(1, 10));
for (map<int, int, MyCompare>::iterator it2 = m2.begin(); it2 != m2.end(); ++it2) {
cout << "key = " << (*it2).first << " value = " << it2->second << endl;
}
}
int main() {
test();
system("pause");
return 0;
}
运行结果:
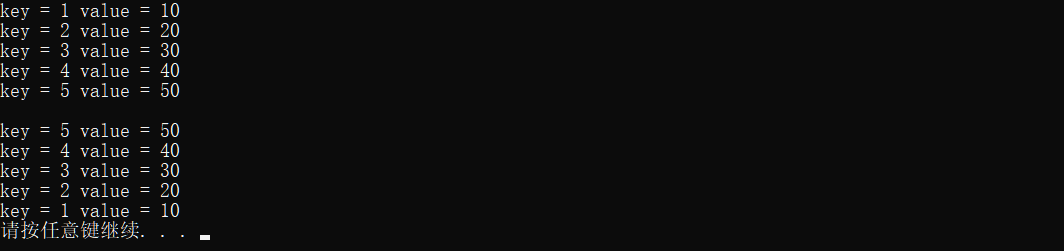
|