11.1 多态基础
11.1.1 为何需要多态行为
#include <iostream>
using namespace std;
class Fish
{
public:
void Swim()
{
cout << "Fish swims! " << endl;
}
};
class Tuna :public Fish
{
public:
void Swim()
{
cout << "Tuna swims! " << endl;
}
};
void MakeFishSwim(Fish& inputFish)
{
inputFish.Swim();
}
int main()
{
Tuna myDinner;
myDinner.Swim();
MakeFishSwim(myDinner);
return 0;
}
结果如下: Tuna swims! Fish swims! 理想情况下,用户希望Tuna对象表现出金枪鱼的行为,即便通过外部函数的Fish参数调用Swim()时亦如此。因此,在MakeFishSwim(Fish& inputFish)函数中调用inputFish.Swim()时,用户希望执行的是Tuna::Swim()。要实现这种多态行为,可将Fish::Swim()声明为虚函数。
11.1.2 使用虚函数实现多态行为
调用语法:
class Base
{
virtual ReturnType FunctionName (Parameter List);
};
class Derived
{
ReturnType FunctionName (Parameter List);
};
通过使用关键字 virtual,可确保编译器调用覆盖版本。也就是说,如果 Swim( )被声明为虚函数,则将参数 myFish(其类型为 Fish&)设置为一个 Tuna 对象时, myFish.Swim( )将执行 Tuna::Swim( )。 示例如下:
#include <iostream>
using namespace std;
class Fish
{
public:
virtual void Swim()
{
cout << "Fish swims! " << endl;
}
};
class Tuna :public Fish
{
public:
void Swim()
{
cout << "Tuna swims! " << endl;
}
};
void MakeFishSwim(Fish& inputFish)
{
inputFish.Swim();
}
int main()
{
Tuna myDinner;
myDinner.Swim();
MakeFishSwim(myDinner);
return 0;
}
总结:多态就是将派生类对象视为基类对象,并执行派生类的 Swim( )实现。
11.1.3 为何需要构造虚函数
对于使用new在自由存储区中实例化的派生类对象,如果将其赋给基类指针,并通过该指针调用delete,将不会调用派生类的析构函数。这可能导致资源未释放、内存泄露等问题。 示例如下:
#include <iostream>
using namespace std;
class Fish
{
public:
Fish()
{
cout << "Constructed Fish" << endl;
}
~Fish()
{
cout << "Destroyed Fish" << endl;
}
};
class Tuna :public Fish
{
public:
Tuna()
{
cout << "Constructed Tuna" << endl;
}
~Tuna()
{
cout << "Destroyed Tuna" << endl;
}
};
void DeleteFishMemory(Fish* pFish)
{
delete pFish;
}
int main()
{
cout << "Allocating a Tuna on the free store:" << endl;
Tuna* pTuna = new Tuna;
cout << "Deleting the Tuna: "<< endl ;
DeleteFishMemory(pTuna);
cout <<" Instantiating a Tuna on the stack:" << endl;
Tuna myDinner;
cout << "Automatic destruction as it goes out of scope: " << endl;
return 0;
}
结果如下: Allocating a Tuna on the free store: Constructed Fish Constructed Tuna Deleting the Tuna: Destroyed Fish //仅仅释放了基类对象 Instantiating a Tuna on the stack: Constructed Fish Constructed Tuna Automatic destruction as it goes out of scope: Destroyed Tuna Destroyed Fish
要避免上述问题,可将基类中的析构函数声明为虚函数:
#include <iostream>
using namespace std;
class Fish
{
public:
Fish()
{
cout << "Constructed Fish" << endl;
}
virtual ~Fish()
{
cout << "Destroyed Fish" << endl;
}
};
class Tuna :public Fish
{
public:
Tuna()
{
cout << "Constructed Tuna" << endl;
}
~Tuna()
{
cout << "Destroyed Tuna" << endl;
}
};
void DeleteFishMemory(Fish* pFish)
{
delete pFish;
}
int main()
{
cout << "Allocating a Tuna on the free store:" << endl;
Tuna* pTuna = new Tuna;
cout << "Deleting the Tuna: "<< endl ;
DeleteFishMemory(pTuna);
cout <<" Instantiating a Tuna on the stack:" << endl;
Tuna myDinner;
cout << "Automatic destruction as it goes out of scope: " << endl;
return 0;
}
结果如下: Allocating a Tuna on the free store: Constructed Fish Constructed Tuna Deleting the Tuna: Destroyed Tuna Destroyed Fish Instantiating a Tuna on the stack: Constructed Fish Constructed Tuna Automatic destruction as it goes out of scope: Destroyed Tuna Destroyed Fish
11.1.4 虚函数的工作原理——理解虚函数表
在编译时,编译器将为Base和Derived类都将有自己的虚函数表(VFT)。实例化这些类的对象时,将创建一个隐藏的指针(VFT*),它指向相应的VFT,可将VFT视为一个包含函数指针的静态数组,其中每个指针都指向相应的虚函数实现。 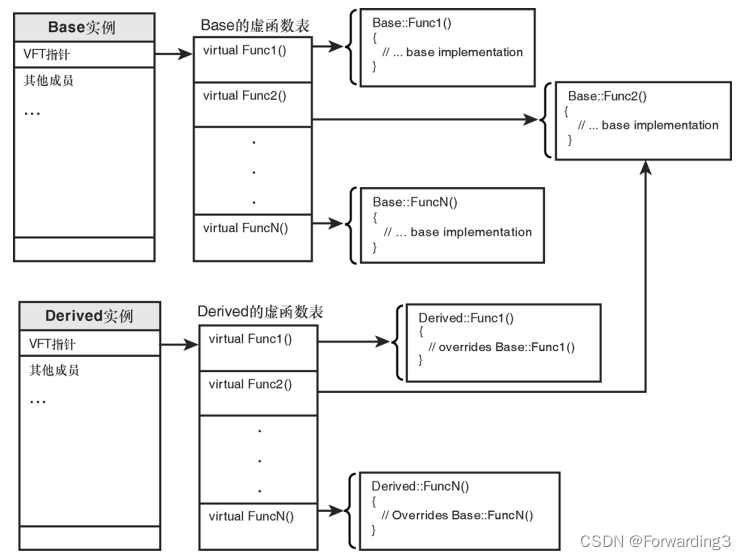 如上图所示,Derived派生类中除了Func2()外,其它虚函数都有Derived类的本地虚函数实现,Derived 没有覆盖Base::Func2( ),因此相应的函数指针指向 Base 类的 Func2( )实现。
使用sizeof证明虚函数表指针存在
#include <iostream>
using namespace std;
class SimpleClass
{
int a, b;
public:
void DoSomething() { }
};
class Base
{
int a, b;
public:
virtual void DoSomething() { }
};
int main()
{
cout << "sizeof (SimpleClass) = " << sizeof(SimpleClass) << endl;
cout << "sizeof (Base) ="<< sizeof (Base) << endl ;
return 0;
}
结果如下: sizeof (SimpleClass) = 8 sizeof (Base) =16 添加关键字virtual带来的影响是,编译器将为Base类生成一个虚函数表,并为其虚函数表指针预留空间。
11.1.5 抽象基类和纯虚函数
抽象基类:不能实例化的基类,这样的基类只有一个用途,那就是从它派生出其他类。要创建抽象基类,可声明纯虚函数。 语法如下:
class AbstractBase
{
public:
virtual void DoSomething() = 0;
};
改声明告诉编译器,AbstractBase的派生类必须实现方法DoSomething() 示例代码:
#include <iostream>
using namespace std;
class Fish
{
public:
virtual void Swim() = 0;
};
class Tuna :public Fish
{
public:
void Swim()
{
cout << "Tuna swims fast in the sea! " << endl;
}
};
class Carp :public Fish
{
public:
void Swim()
{
cout << "Carp swims slow in the lake! " << endl;
}
};
void MakeFishSwim(Fish& inputFish) {
inputFish.Swim();
}
int main()
{
Carp myLunch;
Tuna myDinner;
MakeFishSwim(myLunch);
MakeFishSwim(myDinner);
return 0;
}
结果如下: Carp swims slow in the lake! Tuna swims fast in the sea! 编译器不允许创建抽象基类(ABC)Fish的实例,但是抽象基类可以作为指针或者引用的类型输入函数。
11.2 使用虚继承解决菱形问题
按照如下关系图实例化一个鸭嘴兽的类: 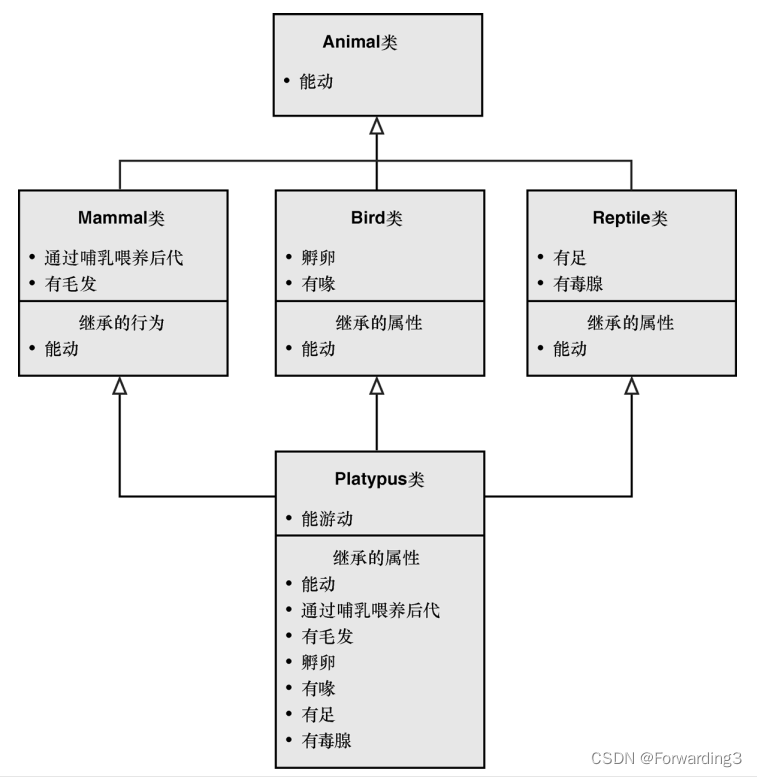 示例代码如下:
#include <iostream>
using namespace std;
class Animal
{
public:
Animal()
{
cout << "Animal constructor" << endl;
}
int age;
};
class Mammal :public Animal
{
};
class Bird :public Animal
{
};
class Reptile :public Animal
{
};
class Platypus:public Mammal, public Bird, public Reptile
{
public:
Platypus()
{
cout << "Platypus constructor" << endl;
}
};
int main()
{
Platypus duckBilledP;
return 0;
}
结果如下: Animal constructor Animal constructor Animal constructor Platypus constructor 由于采用了多继承,且鸭嘴兽的全部三个基类都是从Animal类派生而来的,因此自动创建了3个Animal实例。 下面采用虚继承方式解决这个问题:
#include <iostream>
using namespace std;
class Animal
{
public:
Animal()
{
cout << "Animal constructor" << endl;
}
int age;
};
class Mammal :public virtual Animal
{
};
class Bird :public virtual Animal
{
};
class Reptile :public virtual Animal
{
};
class Platypus final:public Mammal, public Bird, public Reptile
{
public:
Platypus()
{
cout << "Platypus constructor" << endl;
}
};
int main()
{
Platypus duckBilledP;
duckBilledP.age = 25;
return 0;
}
结果如下: Animal constructor Platypus constructor 从 Animal 类派生 Mammal、Bird 和 Reptile 类时,使用了关键字 virtual,这样 Platypus 继承这些类时,每个 Platypus 实例只包含一个 Animal 实例。同时,使用了关键字 final 以禁止将 Platypus 类用作基类。
11.4 使用final来禁止覆盖函数
class Tuna :public Fish
{
public:
void Swim() override final
{
cout << "Tuna swims! " << endl;
}
};
class BluefinTuna final : public Tuna
{
public:
void Swim()
{
}
};
|