常用算术生成算法
学习目标:
注意:
- 算术生成算法属于小型算法,使用时包含的头文件为
#include <numeric>
算法简介:
-
accumulate // 计算容器元素累计总和 -
fill // 向容器中添加元素
1. accumulate
功能描述:
函数原型:
-
accumulate(iterator beg, iterator end, value); 返回一个int型变量 // 计算容器元素累计总和 // beg 开始迭代器 // end 结束迭代器 // value 起始累加值。一般都是从0开始累加
#include<iostream>
using namespace std;
#include<vector>
#include<numeric>
#include<algorithm>
void test01()
{
cout << "针对内置数据类型" << endl;
vector<int> v1;
for (int i = 1; i <=100; i++) {
v1.push_back(i);
}
// 不用accumulate算法,得自己写个for循环去统计容器内各元素累加和
cout << "如果不用accumulate算法,得自己写个for循环去统计容器内各元素累加和" << endl;
int normal_sum1 = 0;
for (int i = 0; i < 100; i++) {
normal_sum1 = normal_sum1 + v1[i];
}
cout <<"normal_sum1 =" << normal_sum1 << endl<<endl<<endl;
int accumulate_sum1 = accumulate(v1.begin(), v1.end(), 0);
cout << "运用accumulate算法直接一步到位:" << endl;
cout << "accumulate_sum1="<< accumulate_sum1 << endl;
}
// 针对自定义数据类型
class Person {
public:
Person(string name, int age) :m_Name(name), m_Age(age) {
}
int m_Age;
string m_Name;
};
int main() {
test01();
cout << endl << endl << endl;
system("pause");
return 0;
}
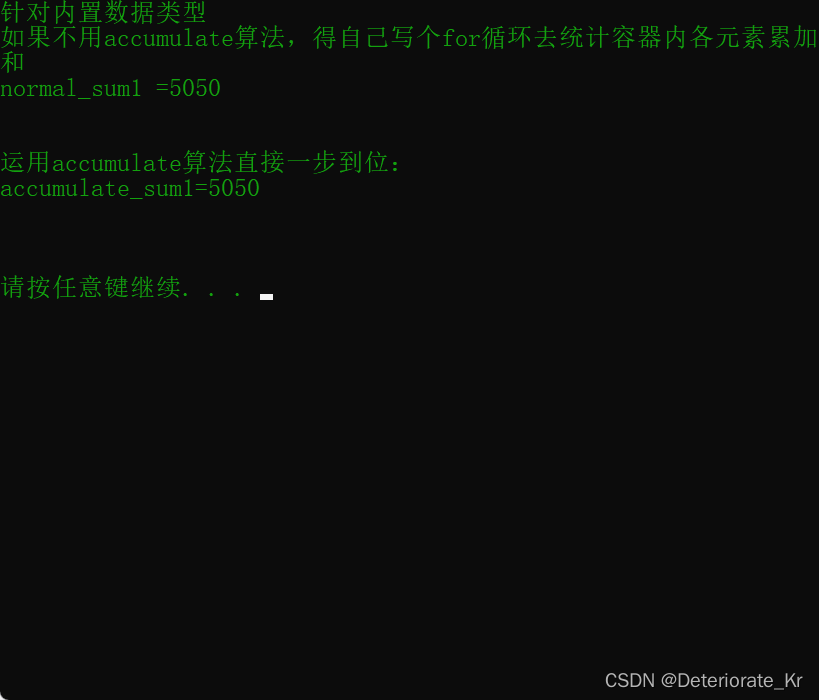
总结 :accumulate使用时头文件注意是 numeric,这个算法很实用
由于所学知识有限,暂时不知道accumulate算法针对自定义数据类型怎么写
2. fill
功能描述:
函数原型:
#include<iostream>
using namespace std;
#include<vector>
#include<numeric>
#include<algorithm>
void Myprint1(int n) {
cout << n << " ";
}
bool Mycompare1(int a, int b) {
return a > b;
}
class Myreplace1 {
public:
bool operator()(int n) {
return n > 66;
}
};
class Myreplace2 {
public:
bool operator()(int n) {
return n < 10;
}
};
void test01()
{
cout << "针对内置数据类型" << endl;
vector<int> v1;
v1.push_back(10);
v1.push_back(201);
v1.push_back(3);
v1.push_back(666);
v1.push_back(58);
v1.push_back(79);
v1.push_back(67);
v1.push_back(6);
v1.push_back(6);
cout << "v1里的元素如下:" << endl;
for_each(v1.begin(), v1.end(), Myprint1); cout << endl << endl;
cout << "将[v1.begin()到v1.end()-1)这个区间里的元素改为666" << endl;
fill(v1.begin(), v1.end() - 1, 666);
for_each(v1.begin(), v1.end(), Myprint1); cout << endl << endl;
cout << "将[v1.begin()到v1.end()-3]这个区间里的元素改为888" << endl;
fill(v1.begin(), v1.end()-3, 888);
for_each(v1.begin(), v1.end(), Myprint1); cout << endl << endl;
}
// 针对自定义数据类型
class Person {
public:
Person(string name, int age) :m_Name(name), m_Age(age) {
}
int m_Age;
string m_Name;
};
void Myprint2(Person p) {
cout << "姓名:" << p.m_Name << " "
<< "年龄:" << p.m_Age << endl;
}
void test02() {
cout << "针对自定义数据类型" << endl;
vector<Person> v2;
//创建数据
Person p1("aaa", 10);
Person p2("bbb", 5);
Person p3("ccc", 60);
Person p4("ddd", 30);
Person p5("grep", 3);
Person p6("awk", 200);
Person p7("sed", 105);
Person p8("Shell", 3060);
v2.push_back(p1);
v2.push_back(p2);
v2.push_back(p3);
v2.push_back(p4);
v2.push_back(p5);
v2.push_back(p6);
cout << "v2里的元素如下:" << endl;
for_each(v2.begin(), v2.end(), Myprint2); cout << endl ;
cout << "p7的姓名:" << p7.m_Name << " "
<< "p7的年龄:" << p7.m_Age << endl << endl;
cout << "先将[v2.begin(),v2.end()-2)这个区间里的对象全改为p7" << endl;
fill(v2.begin(), v2.end() - 2, p7);
for_each(v2.begin(), v2.end(), Myprint2); cout << endl << endl;
cout << "p3的姓名:" << p3.m_Name << " "
<< "p3的年龄:" << p3.m_Age << endl << endl;
cout << "再将[v2.begin(),v2.end()-1)这个区间里的对象全改为p3" << endl;
fill(v2.begin(), v2.end() - 1, p3);
for_each(v2.begin(), v2.end(), Myprint2); cout << endl << endl;
}
int main() {
test01();
test02();
cout << endl << endl << endl;
system("pause");
return 0;
}
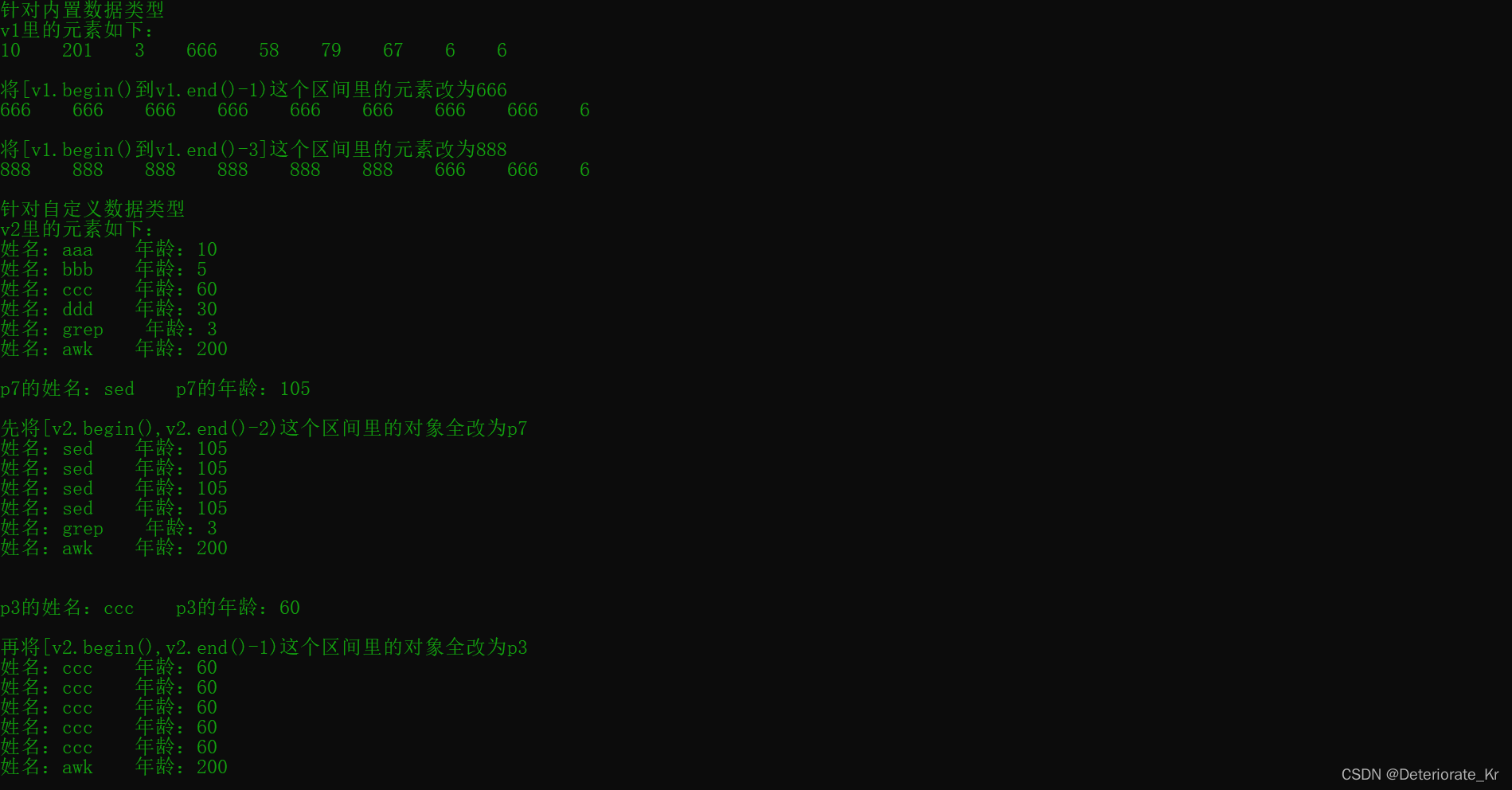 总结:利用fill可以将容器区间内元素填充为 指定的值
|