mongodb 的安装
在ubuntu系统上安装教程
?mongodb服务端的启动
启动和停用mongodb?server
-
启动: sudo service mongod start -
停止: sudo service mongod stop -
重启: sudo service mongod restart
查看启动状态:sudo service mongod status 或者 ps aux | grep mongod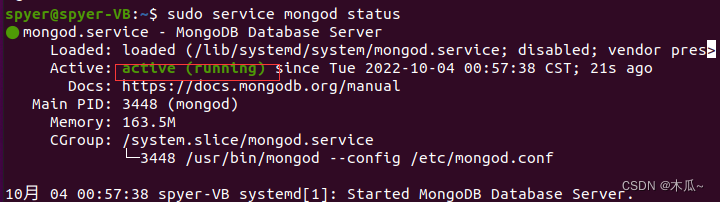
启动和停用 mongodb clients
mongosh的命令使用
-
对数据库的操作
- 查看当前正在使用的数据库:db
- 查看所有的数据库:show dbs? 或者 show databases
- 切换数据库:use db_name? ? ? ?其中:db_name为show dbs后返回的数据库名
- 删除当前的数据库:db.dropDatabase()
-
对collection的操作
- 手动创建集合:格式: db.createCollection(name,options)
eg.?? db.createCollection("first_collection")
????????db.createCollection("first_collection", { capped : true, size : 10 } )
????????参数capped:默认值为false表示不设置上限,值为true表示设置上限
????????参数size:集合所占用的字节数。 当capped值为true时,需要指定此参数,表示上限大小,当文档达到上限时, 会将之前的数据覆盖,单位为字节.
- 检查集合是否设定上限: db.<集合名>.isCapped()
- 查看集合:show collections
- 删除集合:db.<集合名称>.drop()
MongoDB的常见数据类型
- Object ID: 文档或数据的ID,主键
- String: 字符串,最常用,必须是有效的UTF-8
- Boolean: 存储一个布尔值,true或false
- Integer: 整数可以是32位或64位,这取决于服务器
- Double: 浮点数
- Arrays: 数组/列表
- Object: mongodb中的一条数据/文档,即文档嵌套文档
- Null: 存储null值
- Timestamp: 时间戳,表示从1970-1-1到现在的总秒数
- Date: 存储当前日期或时间的UNIX时间格式
【知识点】
- 每条文档数据都有一个id属性,保证每条文档数据的唯一性,mongodb默认使用id作为主键
- 可以手动设置id的值,如果没有提供,那么MongoDB为每条文档数据提供了一个独特的id, 类型为objectID
- objectID是一个12字节的十六进制数,每个字节两位,一共是24位的字符串:
- 前4个字节为当前时间戳
- 接下来3个字节的机器ID
- 接下来的2个字节中MongoDB的服务进程id
- 最后3个字节是简单的增量值
mongodb的增、删、改、查命令
增加(插入)数据
mongodb提供了两个插入命令用于新增数据,如果插入数据时,集合(collection)不存在,则会插入新数据时候创建新的集合。并且插入文档时,如果不指定_id参数,MongoDB会为文档自动分配一个唯一的ObjectId。
例子:
插入一条文档数据
use sample_mflix
db.movies.insertOne(
{
title: "The Favourite",
genres: [ "Drama", "History" ],
runtime: 121,
rated: "R",
year: 2018,
directors: [ "Yorgos Lanthimos" ],
cast: [ "Olivia Colman", "Emma Stone", "Rachel Weisz" ],
type: "movie"
}
)
插入多条文档数据:
use sample_mflix
db.movies.insertMany([
{
title: "Jurassic World: Fallen Kingdom",
genres: [ "Action", "Sci-Fi" ],
runtime: 130,
rated: "PG-13",
year: 2018,
directors: [ "J. A. Bayona" ],
cast: [ "Chris Pratt", "Bryce Dallas Howard", "Rafe Spall" ],
type: "movie"
},
{
title: "Tag",
genres: [ "Comedy", "Action" ],
runtime: 105,
rated: "R",
year: 2018,
directors: [ "Jeff Tomsic" ],
cast: [ "Annabelle Wallis", "Jeremy Renner", "Jon Hamm" ],
type: "movie"
}
])
查询结果可以用:
db.movies.find( { title: "The Favourite" } )
db.movies.find( {} )
删除数据
例子:
删除collection的所有文档数据
use sample_mflix
db.movies.deleteMany({})
删除集合中,所有符合title为 "Titanic"条件的数据
use sample_mflix
db.movies.deleteMany( { title: "Titanic" } )
删除集合中,第一条符合title为 "Titanic"条件的数据
use sample_mflix
db.movies.deleteOne( { title: "Titanic" } )
修改数据
?【注意】:db.collection.update() 已经被弃用了
字段更新运算符(Field Update Operators)
Name | Description |
---|
$currentDate | Sets the value of a field to current date, either as a Date or a Timestamp. | $inc | Increments the value of the field by the specified amount. | $min | Only updates the field if the specified value is less than the existing field value. | $max | Only updates the field if the specified value is greater than the existing field value. | $mul | Multiplies the value of the field by the specified amount. | $rename | Renames a field. | $set | Sets the value of a field in a document. | $setOnInsert | Sets the value of a field if an update results in an insert of a document. Has no effect on update operations that modify existing documents. | $unset | Removes the specified field from a document. |
数组更新运算符
Name | Description |
---|
$ | Acts as a placeholder to update the first element that matches the query condition. | $[] | Acts as a placeholder to update all elements in an array for the documents that match the query condition. | $[<identifier>] | Acts as a placeholder to update all elements that match the?arrayFilters ?condition for the documents that match the query condition. | $addToSet | Adds elements to an array only if they do not already exist in the set. | $pop | Removes the first or last item of an array. | $pull | Removes all array elements that match a specified query. | $push | Adds an item to an array. | $pullAll | Removes all matching values from an array. |
查询数据
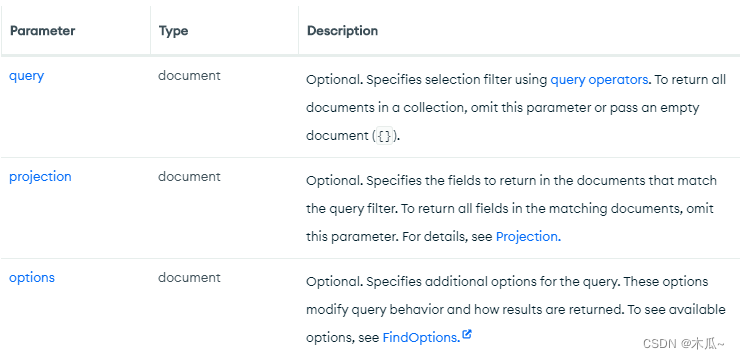
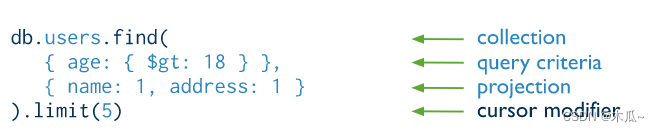
?例子:
插入一条数据
db.movies.insertMany([{
"name" : { "first" : 'zhou', "last" : "changping" },
"birth" : '2022-09-09',
"contribs" : [ 'document', 'help other people' ],
"awards" : [
{ "award" : 'nobie price', "year": '2030-10-3' }
]
},
{
"name" : { "first" : 'zhou', "last" : "changping second" },
"birth" : '2022-09-09',
"contribs" : [ 'build a network', 'help other people' ],
"awards" : [
{ "award" : 'nobie price', "year": '2030-10-3' }
]
}]
)
查询数据,这里使用了点符号方式,查询name.last 为changping的,完全匹配
db.movies.find({'name.last': 'changping'})
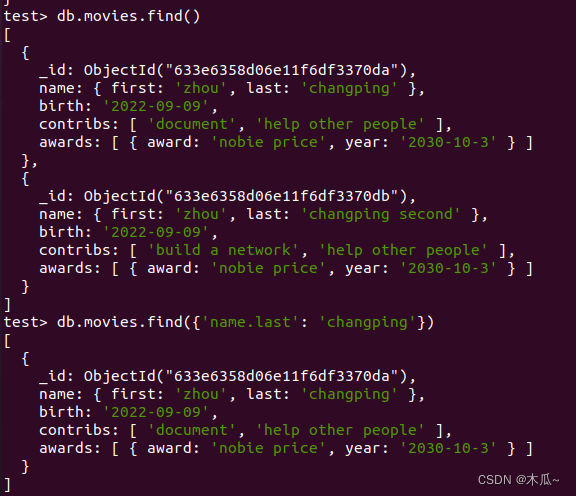
比较操作符
例子:
插入数据
db.inventory.insertMany( [
{ "item": "Pens", "quantity": 350, "tags": [ "school", "office" ] },
{ "item": "Erasers", "quantity": 15, "tags": [ "school", "home" ] },
{ "item": "Maps", "tags": [ "office", "storage" ] },
{ "item": "Books", "quantity": 5, "tags": [ "school", "storage", "home" ] }
] )
以下查询从集合中选择数量不等于5或15的所有文档。或还匹配没有数量字段的文档。
db.inventory.find( { quantity: { $nin: [ 5, 15 ] } }, { _id: 0 } )
返回结果如下:
{ item: 'Pens', quantity: 350, tags: [ 'school', 'office' ] },
{ item: 'Maps', tags: [ 'office', 'storage' ] }
逻辑操作符
其中:
-
$nor表示的是返回都不匹配组合条件的文档,包括字段不存在。
-
格式:
-
$not格式:{ field: { $not: { <operator-expression> } } } -
其它三个格式:{ <操作符>: [ { <expression1> }, { <expression2> }, ... { <expressionN> } ] }
-
例子:
db.inventory.find( { $nor: [ { 'price': 1.99 }, { 'sale': true } ] } )
解释:此查询将返回以下所有文档:1.两个字段不存在,2. 一个字段不存在,一个不符合条件,3. 两个字段存在,但都不符合条件
??db.inventory.find( { $nor: [ { 'price': 1.99 }, { 'price': { $exists: false } },{ 'sale': true }, { 'sale': { $exists: false } } ] } )
??解释:此查询将返回以下所有文档:两字段必须存在,但不符合条件的文档
??包含price 字段但值不等于1.99和包含sale 字段但值不等于true
??db.inventory.find( { item: { $not: /^p.*/ } } )
??db.inventory.find( { item: { $not: { $regex: "^p.*" } } } )
??db.inventory.find( { item: { $not: { $regex: /^p.*/ } } } )
??解释:这三个是一样的查询结果,此查询将返回一下所有文档
-
包含值不等于1.99的price字段和值不等于true的sale字段,或 -
包含其值不等于1.99的price字段,但不包含sale字段或 -
不包含price字段,但包含sale字段其值不等于true或 -
不包含price字段,也不包含sale字段 -
包含item字段,但是值不是以p开头或 -
不包含item字段的
元素操作符
权限用户管理
创建用户
创建超级管理用户
use admin
db.createUser({"user":"admin","pwd":"password","roles":["root"]})
成功返回{ok:1}

创建普通用户
use admin
db.createUser({"user":"python","pwd":"python","roles":[{role: "readWrite", db: "admin"}]})
db.createUser({"user":"read","pwd":"read","roles":[{role: "readAnyDatabase", db: "admin"}]})
列出所有用户
use admin
db.system.users.find()
列出当前数据库的所有用户
show users
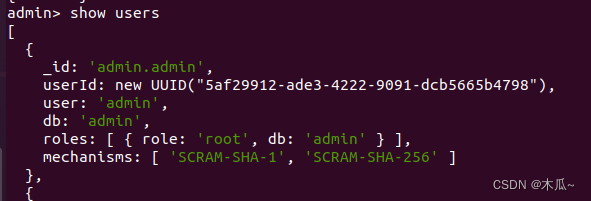
配置文件(/etc/mongod.conf)
?【注意】:如果想要让权限管理限制生效,就必须配置/etc/mongod.conf文件,设置?security.authorization为enabled才行。
security:
authorization: enabled
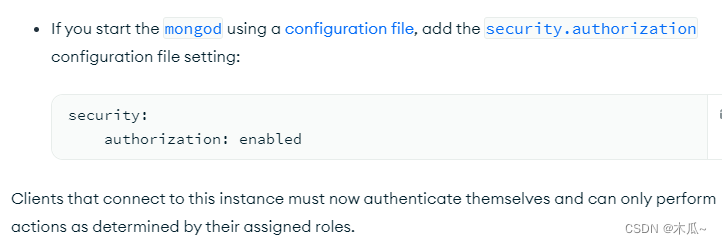
登录方式
打开mongo shell的时候,输入用户名和密码
mongosh "mongodb://admin:password@localhost:27017/admin"
进入shell时,不输入,进入shell之后使用权限管理
db.auth( "username", "password" )
或者
db.auth( "username", passwordPrompt() )
删除用户
db.runCommand(
{
dropUser: "<user>",
writeConcern: { <write concern> },
comment: <any>
}
)
已经存在的用户添加新权限grantRolesToUser
语法格式
db.runCommand(
{
grantRolesToUser: "<user>",
roles: [ <roles> ],
writeConcern: { <write concern> },
comment: <any>
}
)
例子:
?假如原始用户python的权限如下
{
_id: 'admin.python',
userId: new UUID("ccae0784-7376-4817-8aba-9918ad38d615"),
user: 'python',
db: 'admin',
roles: [ { role: 'read', db: 'dbname' } ],
mechanisms: [ 'SCRAM-SHA-1', 'SCRAM-SHA-256' ]
}
修改权限
db.runCommand({grantRolesToUser: "python", roles: [{role:"read", db:"dbname"}, "readWrite"]})
修改后结果如下:
{
_id: 'admin.python',
userId: new UUID("ccae0784-7376-4817-8aba-9918ad38d615"),
user: 'python',
db: 'admin',
roles: [
{ role: 'read', db: 'dbname' },
{ role: 'readWrite', db: 'admin' }
],
mechanisms: [ 'SCRAM-SHA-1', 'SCRAM-SHA-256' ]
}
?以下是权限开启后,通过python用户更新dbname数据库时,就会报权限不足的错误,因为从上面可知,python用户对dbname数据库只有只读权限。
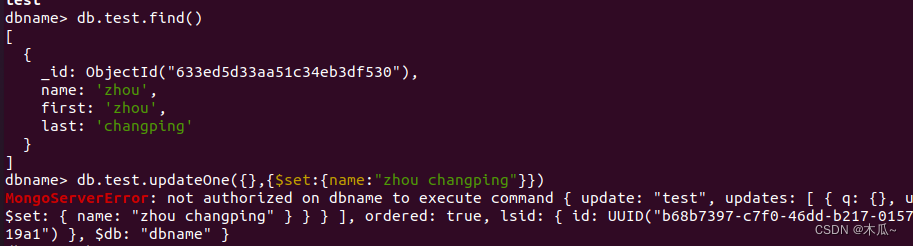
?
|