《建造我的Android技能塔之沙粒二:Handler机制》
Handler机制
一、ThreadLocal
-
THreadLocal是一个线程内部的数据存储类,通过它可以在指定的线程中获取数据,数据存储以后,只有在指定线程中可以获取到存储的数据,对于其他线程 来说,则无法获取到数据。 -
使用场景: ① 当某些数据是以线程为作用域并且不同的线程具有不同的Looper,这时候通过ThreadLocal就可以轻松实现Looper在线程中的存取。 ②复杂逻辑下对象的传递,比如监听器的传递,有些时候一个线程中的任务过于复杂,这可能表现为函数调用栈比较深以及代码入口的多样性,在这种情况下,有需要监听器能够贯穿整个线程的执行过程,这个时候就可以采用ThreadLocal,采用ThreadLocal可以让监听器作为线程内的全局对象而存在,在线程内部只需要get就可以获取到监听器。 -
示例:
public static final String TAG = "StudyTest";
private ThreadLocal<Boolean> mThreadLocal = new ThreadLocal<Boolean>();
@Test
public void useAppContext() {
mThreadLocal.set(true);
Log.d(TAG,"[ThreadLocal#main] mThreadLocal = " + mThreadLocal.get() );
new Thread("Thread1"){
@Override
public void run() {
mThreadLocal.set(false);
Log.d(TAG,"[Thread#1] mThreadLocal = " + mThreadLocal.get());
}
}.start();
new Thread("Thread2"){
@Override
public void run() {
Log.d(TAG,"[Thread#2] mThreadLocal = " + mThreadLocal.get());
}
}.start();
}
D/StudyTest: [ThreadLocal#main] mThreadLocal = true
D/StudyTest: [Thread#1] mThreadLocal = false
D/StudyTest: [Thread#2] mThreadLocal = null
从以上可以看出,虽然在不同线程中访问的是同一个ThreadLocal对象,但是他们通过ThreadLocal获取的值却是不一样的。
? ThreadLocal之所以有这么神奇的效果,是因为不同线程访问同一个THreadLocal的get方法,ThreadLocal内部会从各自的线程中取出一个数组,然后再从数组中根据当前ThreadLocal的索引去查找对应的value的值。
? 显然不同的线程中的数组是不同的,这就是为什么通过ThreadLocal的索引去查找出对应的value值。
-
ThreadLocal内部实现 ThreadLocal 是一个泛型类。 ThreadLocal set方法
public void set(T value) {
Thread t = Thread.currentThread();
ThreadLocalMap map = getMap(t);
if (map != null)
map.set(this, value);
else
createMap(t, value);
}
ThreadLocalMap set方法
private void set(ThreadLocal<?> key, Object value) {
Entry[] tab = table;
int len = tab.length;
int i = key.threadLocalHashCode & (len-1);
for (Entry e = tab[i];
e != null;
e = tab[i = nextIndex(i, len)]) {
ThreadLocal<?> k = e.get();
if (k == key) {
e.value = value;
return;
}
if (k == null) {
replaceStaleEntry(key, value, i);
return;
}
}
tab[i] = new Entry(key, value);
int sz = ++size;
if (!cleanSomeSlots(i, sz) && sz >= threshold)
rehash();
}
ThreadLocal get方法
public T get() {
Thread t = Thread.currentThread();
ThreadLocalMap map = getMap(t);
if (map != null) {
ThreadLocalMap.Entry e = map.getEntry(this);
if (e != null) {
@SuppressWarnings("unchecked")
T result = (T)e.value;
return result;
}
}
return setInitialValue();
}
private T setInitialValue() {
T value = initialValue();
Thread t = Thread.currentThread();
ThreadLocalMap map = getMap(t);
if (map != null)
map.set(this, value);
else
createMap(t, value);
return value;
}
void createMap(Thread t, T firstValue) {
t.threadLocals = new ThreadLocalMap(this, firstValue);
}
二、 消息队列工作原理
? 消息队列指的是 MessageQueue,MessageQueue主要包含两个操作:插入和读取。 读取操作本身会伴随着删除操作,插入和读取对应的方法分别是enqueueMessage()和next()方法的实现。
MessageQueue实际上是一个单链表。
enqueueMessage 方法
boolean enqueueMessage(Message msg, long when) {
if (msg.target == null) {
throw new IllegalArgumentException("Message must have a target.");
}
synchronized (this) {
if (msg.isInUse()) {
throw new IllegalStateException(msg + " This message is already in use.");
}
if (mQuitting) {
IllegalStateException e = new IllegalStateException(
msg.target + " sending message to a Handler on a dead thread");
Log.w(TAG, e.getMessage(), e);
msg.recycle();
return false;
}
msg.markInUse();
msg.when = when;
Message p = mMessages;
boolean needWake;
if (p == null || when == 0 || when < p.when) {
msg.next = p;
mMessages = msg;
needWake = mBlocked;
} else {
needWake = mBlocked && p.target == null && msg.isAsynchronous();
Message prev;
for (;;) {
prev = p;
p = p.next;
if (p == null || when < p.when) {
break;
}
if (needWake && p.isAsynchronous()) {
needWake = false;
}
}
msg.next = p;
prev.next = msg;
}
if (needWake) {
nativeWake(mPtr);
}
}
return true;
}
next方法
Message next() {
final long ptr = mPtr;
if (ptr == 0) {
return null;
}
int pendingIdleHandlerCount = -1;
int nextPollTimeoutMillis = 0;
for (;;) {
if (nextPollTimeoutMillis != 0) {
Binder.flushPendingCommands();
}
nativePollOnce(ptr, nextPollTimeoutMillis);
synchronized (this) {
final long now = SystemClock.uptimeMillis();
Message prevMsg = null;
Message msg = mMessages;
if (msg != null && msg.target == null) {
do {
prevMsg = msg;
msg = msg.next;
} while (msg != null && !msg.isAsynchronous());
}
if (msg != null) {
if (now < msg.when) {
nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE);
} else {
mBlocked = false;
if (prevMsg != null) {
prevMsg.next = msg.next;
} else {
mMessages = msg.next;
}
msg.next = null;
if (DEBUG) Log.v(TAG, "Returning message: " + msg);
msg.markInUse();
return msg;
}
} else {
nextPollTimeoutMillis = -1;
}
if (mQuitting) {
dispose();
return null;
}
if (pendingIdleHandlerCount < 0
&& (mMessages == null || now < mMessages.when)) {
pendingIdleHandlerCount = mIdleHandlers.size();
}
if (pendingIdleHandlerCount <= 0) {
mBlocked = true;
continue;
}
if (mPendingIdleHandlers == null) {
mPendingIdleHandlers = new IdleHandler[Math.max(pendingIdleHandlerCount, 4)];
}
mPendingIdleHandlers = mIdleHandlers.toArray(mPendingIdleHandlers);
}
for (int i = 0; i < pendingIdleHandlerCount; i++) {
final IdleHandler idler = mPendingIdleHandlers[i];
mPendingIdleHandlers[i] = null;
boolean keep = false;
try {
keep = idler.queueIdle();
} catch (Throwable t) {
Log.wtf(TAG, "IdleHandler threw exception", t);
}
if (!keep) {
synchronized (this) {
mIdleHandlers.remove(idler);
}
}
}
pendingIdleHandlerCount = 0;
nextPollTimeoutMillis = 0;
}
}
next是一个无限循环的方法,若果消息队列中没有消息,那么next方法会一直阻塞在这里。当有新的消息到来时,next方法会返回这条消息并将其从单链表中移除。
三、Looper工作原理
? Looper负责不停的从MessageQueue中查看是否有新消息,如果有新消息就会立刻处理,否则就一直阻塞在那里。下面是Looper的构造方法,在Looper创建时,他会自动创建一个消息队列,然后将当前线程的对象保存起来。
private Looper(boolean quitAllowed) {
mQueue = new MessageQueue(quitAllowed);
mThread = Thread.currentThread();
}
? 创建Looper
new Thread("Thread2"){
@Override
public void run() {
Looper.prepare();
Handler handler = new Handler();
Looper.loop();
}
}.start();
? Looper除了prepare方法外,还提供了prepareMainLooper方法,这个方法主要是给主线程也就是ActivityThread创建Looper使用的。其本质也是prepare方法。
? 由于主线程的Looper比较特殊,所以Looper提供了一个getMainLooper方法,通过它可以在任何地方获取到主线程的Looper。
? Looper退出方法:1. quit: 直接退出;2.quitSafely :设定一个退出标记,然后把消息队列中的已有消息处理完毕后才安全的退出。
? Looper最重要的是loop方法,只有调用了loop方法后,消息系统才会真正的起作用。
public static void loop() {
final Looper me = myLooper();
if (me == null) {
throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread.");
}
if (me.mInLoop) {
Slog.w(TAG, "Loop again would have the queued messages be executed"
+ " before this one completed.");
}
me.mInLoop = true;
final MessageQueue queue = me.mQueue;
Binder.clearCallingIdentity();
final long ident = Binder.clearCallingIdentity();
final int thresholdOverride =
SystemProperties.getInt("log.looper."
+ Process.myUid() + "."
+ Thread.currentThread().getName()
+ ".slow", 0);
boolean slowDeliveryDetected = false;
for (;;) {
Message msg = queue.next();
if (msg == null) {
return;
}
final Printer logging = me.mLogging;
if (logging != null) {
logging.println(">>>>> Dispatching to " + msg.target + " " +
msg.callback + ": " + msg.what);
}
final Observer observer = sObserver;
final long traceTag = me.mTraceTag;
long slowDispatchThresholdMs = me.mSlowDispatchThresholdMs;
long slowDeliveryThresholdMs = me.mSlowDeliveryThresholdMs;
if (thresholdOverride > 0) {
slowDispatchThresholdMs = thresholdOverride;
slowDeliveryThresholdMs = thresholdOverride;
}
final boolean logSlowDelivery = (slowDeliveryThresholdMs > 0) && (msg.when > 0);
final boolean logSlowDispatch = (slowDispatchThresholdMs > 0);
final boolean needStartTime = logSlowDelivery || logSlowDispatch;
final boolean needEndTime = logSlowDispatch;
if (traceTag != 0 && Trace.isTagEnabled(traceTag)) {
Trace.traceBegin(traceTag, msg.target.getTraceName(msg));
}
final long dispatchStart = needStartTime ? SystemClock.uptimeMillis() : 0;
final long dispatchEnd;
Object token = null;
if (observer != null) {
token = observer.messageDispatchStarting();
}
long origWorkSource = ThreadLocalWorkSource.setUid(msg.workSourceUid);
try {
msg.target.dispatchMessage(msg);
if (observer != null) {
observer.messageDispatched(token, msg);
}
dispatchEnd = needEndTime ? SystemClock.uptimeMillis() : 0;
} catch (Exception exception) {
if (observer != null) {
observer.dispatchingThrewException(token, msg, exception);
}
throw exception;
} finally {
ThreadLocalWorkSource.restore(origWorkSource);
if (traceTag != 0) {
Trace.traceEnd(traceTag);
}
}
if (logSlowDelivery) {
if (slowDeliveryDetected) {
if ((dispatchStart - msg.when) <= 10) {
Slog.w(TAG, "Drained");
slowDeliveryDetected = false;
}
} else {
if (showSlowLog(slowDeliveryThresholdMs, msg.when, dispatchStart, "delivery",
msg)) {
slowDeliveryDetected = true;
}
}
}
if (logSlowDispatch) {
showSlowLog(slowDispatchThresholdMs, dispatchStart, dispatchEnd, "dispatch", msg);
}
if (logging != null) {
logging.println("<<<<< Finished to " + msg.target + " " + msg.callback);
}
final long newIdent = Binder.clearCallingIdentity();
if (ident != newIdent) {
Log.wtf(TAG, "Thread identity changed from 0x"
+ Long.toHexString(ident) + " to 0x"
+ Long.toHexString(newIdent) + " while dispatching to "
+ msg.target.getClass().getName() + " "
+ msg.callback + " what=" + msg.what);
}
msg.recycleUnchecked();
}
}
? loop方法是一个死循环,唯一跳出循环的方式就是MessageQueue的next方法返回了null。当Looper的quit方法呗调用时,Looper就会调用MessageQueue的quit方法或者quitSafely方法来通知消息队列的退出,当消息队列被标记为退出状态时,next方法就会返回null.。也就是说Looper必须退出,否则loop方法无限循环下去。
? loop方法回调用MessageQueue的next方法来获取消息,而next方法是一个阻塞操作,没有消息时,会一直阻塞在那里,也导致loop方法一直阻塞在那里。
? 如果MessageQueue的next方法反悔了消息,Looper就会处理这条消息:msg.target.dispatchMessage(msg),这里的msg.target是发送这条消息的Handler对象,这样Handler发送的消息最终又交给它的dispatchMessage方法来处理了。这里不同的是,Handler的dispatchMessage方法是在创建Handler时所使用的Looper中执行的,这样就成功的将代码逻辑切换到指定线程中去了。
四、Handler工作原理
?
public boolean sendMessageAtTime(@NonNull Message msg, long uptimeMillis) {
MessageQueue queue = mQueue;
if (queue == null) {
RuntimeException e = new RuntimeException(
this + " sendMessageAtTime() called with no mQueue");
Log.w("Looper", e.getMessage(), e);
return false;
}
return enqueueMessage(queue, msg, uptimeMillis);
}
private boolean enqueueMessage(@NonNull MessageQueue queue, @NonNull Message msg,
long uptimeMillis) {
msg.target = this;
msg.workSourceUid = ThreadLocalWorkSource.getUid();
if (mAsynchronous) {
msg.setAsynchronous(true);
}
return queue.enqueueMessage(msg, uptimeMillis);
}
可以发现,Handler发送消息的过程就是想消息队列中插入一条消息,,MessageQueue的next方法就会返回这条消息给Looper,Looper收到消息后就开始处理了,最终消息由Looper交给Handler处理,即Handler的dispatchMessage方法会被调用,这时Handler就进入了处理消息阶段。dispatchMessage消息如下:
public void dispatchMessage(@NonNull Message msg) {
if (msg.callback != null) {
handleCallback(msg);
} else {
if (mCallback != null) {
if (mCallback.handleMessage(msg)) {
return;
}
}
handleMessage(msg);
}
}
Handler处理消息的过程如下:
首先,检查Message的callback是一个Runnable对象,实际上就是Handler的post方法所传递的Runnable参数。handleCallback逻辑如下:
private static void handleCallback(Message message) {
message.callback.run();
}
callback是一个接口:
public interface Callback {
boolean handleMessage(@NonNull Message msg);
}
? 通过callback可采用如下方式来创建Handler对象: Handler h = new Handler(callback);
? CallBack 的作用:可以用来创建一个Handler的实例但并不需要派生Handler的子类。
? 最后,调用Handler的handleMessage方法来处理。Handler处理消息可以归纳为一个流程图:
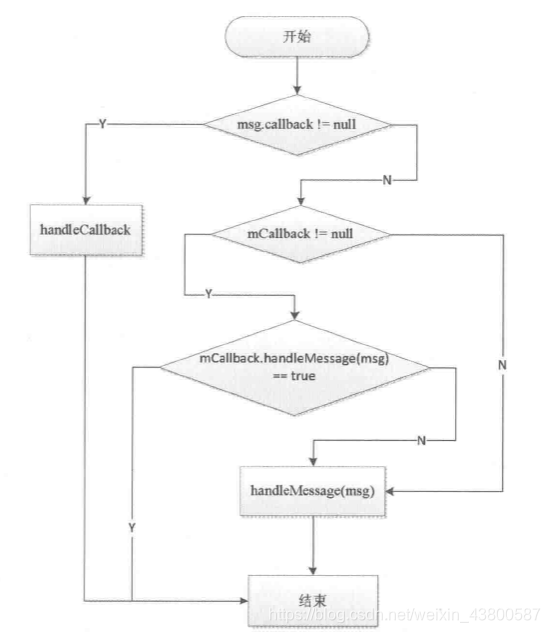
Handler还有个特殊的构造方法——通过一个特定的Looper来构造Handler。
public Handler(@NonNull Looper looper) {
this(looper, null, false);
}
很明显当前线程如果没有Looper的话,就会抛出异常。
public Handler(@Nullable Callback callback, boolean async) {
if (FIND_POTENTIAL_LEAKS) {
final Class<? extends Handler> klass = getClass();
if ((klass.isAnonymousClass() || klass.isMemberClass() || klass.isLocalClass()) &&
(klass.getModifiers() & Modifier.STATIC) == 0) {
Log.w(TAG, "The following Handler class should be static or leaks might occur: " +
klass.getCanonicalName());
}
}
mLooper = Looper.myLooper();
if (mLooper == null) {
throw new RuntimeException(
"Can't create handler inside thread " + Thread.currentThread()
+ " that has not called Looper.prepare()");
}
mQueue = mLooper.mQueue;
mCallback = callback;
mAsynchronous = async;
}
五、主线程的消息循环
? Android中的主线程就是ActivityThread,主线程的入口方法为main,在Main方法中系统会通过Looper.prepareMainLooper()来创建主线程的Looper以及MessageQueue,并通过Looper.loop()来开启主线程的 消息循环。
? 主线程的消息循环开始以后,ActivityThread还需要一个Handler也就是ActivityThread.H,它内部定义了一组消息类型,主要包含了四大组件的启动和停止的过程。
class H extends Handler {
5 public static final int BIND_APPLICATION = 110;
6 @UnsupportedAppUsage
7 public static final int EXIT_APPLICATION = 111;
8 @UnsupportedAppUsage
9 public static final int RECEIVER = 113;
10 @UnsupportedAppUsage
11 public static final int CREATE_SERVICE = 114;
12 @UnsupportedAppUsage
13 public static final int SERVICE_ARGS = 115;
14 @UnsupportedAppUsage
15 public static final int STOP_SERVICE = 116;
16
17 public static final int CONFIGURATION_CHANGED = 118;
18 ...
19 @UnsupportedAppUsage
20 public static final int BIND_SERVICE = 121;
21 @UnsupportedAppUsage
22 public static final int UNBIND_SERVICE = 122;
23 ...
24
25 public static final int EXECUTE_TRANSACTION = 159;
26
27 public static final int RELAUNCH_ACTIVITY = 160;
28 public static final int PURGE_RESOURCES = 161;
29
30 public void handleMessage(Message msg) {
31 if (DEBUG_MESSAGES) Slog.v(TAG, ">>> handling: " + codeToString(msg.what));
32 switch (msg.what) {
33 case BIND_APPLICATION:
34 Trace.traceBegin(Trace.TRACE_TAG_ACTIVITY_MANAGER, "bindApplication");
35 AppBindData data = (AppBindData)msg.obj;
36 handleBindApplication(data);
37 Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
38 break;
39 case EXIT_APPLICATION:
40 if (mInitialApplication != null) {
41 mInitialApplication.onTerminate();
42 }
43 Looper.myLooper().quit();
44 break;
45 case RECEIVER:
46 Trace.traceBegin(Trace.TRACE_TAG_ACTIVITY_MANAGER, "broadcastReceiveComp");
47 handleReceiver((ReceiverData)msg.obj);
48 Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
49 break;
50 case CREATE_SERVICE:
51 Trace.traceBegin(Trace.TRACE_TAG_ACTIVITY_MANAGER, ("serviceCreate: " + String.valueOf(msg.obj)));
52 handleCreateService((CreateServiceData)msg.obj);
53 Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
54 break;
55 case BIND_SERVICE:
56 Trace.traceBegin(Trace.TRACE_TAG_ACTIVITY_MANAGER, "serviceBind");
57 handleBindService((BindServiceData)msg.obj);
58 Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
59 break;
60 case UNBIND_SERVICE:
61 Trace.traceBegin(Trace.TRACE_TAG_ACTIVITY_MANAGER, "serviceUnbind");
62 handleUnbindService((BindServiceData)msg.obj);
63 schedulePurgeIdler();
64 Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
65 break;
66 case SERVICE_ARGS:
67 Trace.traceBegin(Trace.TRACE_TAG_ACTIVITY_MANAGER, ("serviceStart: " + String.valueOf(msg.obj)));
68 handleServiceArgs((ServiceArgsData)msg.obj);
69 Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
70 break;
71 case STOP_SERVICE:
72 Trace.traceBegin(Trace.TRACE_TAG_ACTIVITY_MANAGER, "serviceStop");
73 handleStopService((IBinder)msg.obj);
74 schedulePurgeIdler();
75 Trace.traceEnd(Trace.TRACE_TAG_ACTIVITY_MANAGER);
76 break;
77 case CONFIGURATION_CHANGED:
78 handleConfigurationChanged((Configuration) msg.obj);
79 break;
80
81 ...
82
83 case EXECUTE_TRANSACTION:
84 final ClientTransaction transaction = (ClientTransaction) msg.obj;
85 mTransactionExecutor.execute(transaction);
86 if (isSystem()) {
87
88
89
90 transaction.recycle();
91 }
92
93 break;
94 case RELAUNCH_ACTIVITY:
95 handleRelaunchActivityLocally((IBinder) msg.obj);
96 break;
97 case PURGE_RESOURCES:
98 schedulePurgeIdler();
99 break;
100 }
101 Object obj = msg.obj;
102 if (obj instanceof SomeArgs) {
103 ((SomeArgs) obj).recycle();
104 }
105 if (DEBUG_MESSAGES) Slog.v(TAG, "<<< done: " + codeToString(msg.what));
106 }
107 }
本篇到这就结束啦,这篇内容是今天学习《Android开发艺术探索》做的笔记! 希望不足之处大家多多补充和指正啊!
|