首先你得去支付宝官网下载SDK 这个SDK是一个单独的包,跟 api 'com.alipay.sdk:alipaysdk-android:+@aar’不是同一个。(我是找了很久才明白是分开的)
- 支付宝官方下载SDK链接
- 进去后点击下载开发工具包Android分享SDK包就会自行下载了。
- 然后将下载的SDK包解压,然后进去找到libapshare.jar这个包
- 在你的项目文件res的同级别目录创建一个名为resources的文件夹(注意是创建跟res是同级的文件夹),然后在resources内创建文件夹为libs的子文件夹,然后将libapshare.jar包放进去。
- 然后在点击右键libapshare.jar包找到Add As Library…。点击就会自动给你的项目引用了。
这个时候在build.gradle里面就会生成一条大概这样的一段,就可以正常使用了
implementation files('src\\main\\resources\\libs\\libapshare.jar')
SDK初始化(我是直接全局初始化的,你也可以另行更改) 先在AndroidManifest.xml全局初始化App 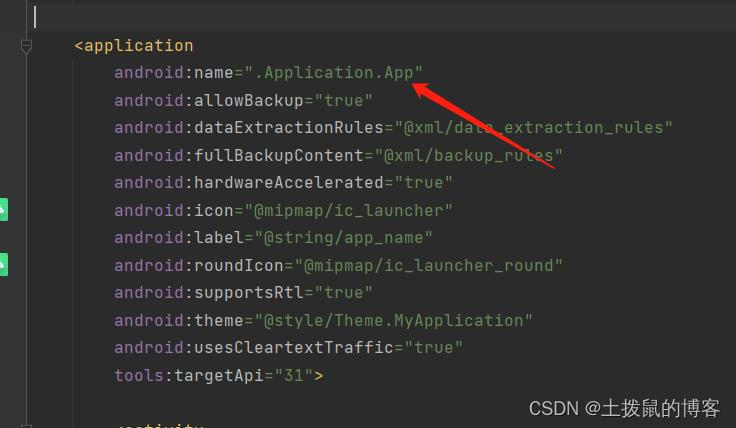 AppConst.AlipayAppId是你的支付宝APPID,请自行更改。
package com.example.myapplication.Application;
import android.app.Application;
import com.alipay.share.sdk.openapi.APAPIFactory;
import com.alipay.share.sdk.openapi.IAPApi;
import com.example.myapplication.Utils.AppConst;
public class App extends Application {
private static IAPApi AlipayApi;
@Override
public void onCreate() {
super.onCreate();
this.registerToAlipay();
}
private void registerToAlipay() {
AlipayApi = APAPIFactory.createZFBApi(getApplicationContext(), AppConst.AlipayAppId, false);
}
public static IAPApi getAlipayApi() {
return AlipayApi;
}
}
支付宝分享工具类
package com.example.myapplication.AlipayApi;
import android.graphics.Bitmap;
import android.widget.Toast;
import com.alipay.share.sdk.openapi.APImageObject;
import com.alipay.share.sdk.openapi.APMediaMessage;
import com.alipay.share.sdk.openapi.APTextObject;
import com.alipay.share.sdk.openapi.APWebPageObject;
import com.alipay.share.sdk.openapi.SendMessageToZFB;
import com.example.myapplication.Application.App;
import com.example.myapplication.Base.BaseActivity;
import java.io.File;
public class AlipayShareUtils {
public static AlipayShareUtils alipayShareUtils;
public enum Singleton {
INSTANCE;
public static AlipayShareUtils getInstance() {
if (alipayShareUtils == null) alipayShareUtils = new AlipayShareUtils();
return new AlipayShareUtils();
}
}
public static void sendWebPageMessage(String title, String desc, String thumbUrl, String url, Boolean timelineChecked) {
APWebPageObject webPageObject = new APWebPageObject();
webPageObject.webpageUrl = url;
APMediaMessage webMessage = new APMediaMessage();
webMessage.title = title;
webMessage.description = desc;
webMessage.mediaObject = webPageObject;
webMessage.thumbUrl = thumbUrl;
SendMessageToZFB.Req webReq = new SendMessageToZFB.Req();
webReq.message = webMessage;
webReq.transaction = buildTransaction("webpage");
if (!isAlipayIgnoreChannel()) {
webReq.scene = timelineChecked ? SendMessageToZFB.Req.ZFBSceneTimeLine : SendMessageToZFB.Req.ZFBSceneSession;
}
App.getAlipayApi().sendReq(webReq);
}
private static void sendTextMessage(String text) {
APTextObject textObject = new APTextObject();
textObject.text = text;
APMediaMessage mediaMessage = new APMediaMessage();
mediaMessage.mediaObject = textObject;
SendMessageToZFB.Req req = new SendMessageToZFB.Req();
req.message = mediaMessage;
App.getAlipayApi().sendReq(req);
}
private static void sendLocalImage(String path) {
File file = new File(path);
if (!file.exists()) {
Toast.makeText(BaseActivity.getContext(), "选择的文件不存在", Toast.LENGTH_SHORT).show();
return;
}
APImageObject imageObject = new APImageObject();
imageObject.imagePath = path;
APMediaMessage mediaMessage = new APMediaMessage();
mediaMessage.mediaObject = imageObject;
SendMessageToZFB.Req req = new SendMessageToZFB.Req();
req.message = mediaMessage;
req.transaction = buildTransaction("image");
App.getAlipayApi().sendReq(req);
}
private static void sendByteImage(Bitmap bmp) {
APImageObject imageObject = new APImageObject(bmp);
APMediaMessage mediaMessage = new APMediaMessage();
mediaMessage.mediaObject = imageObject;
SendMessageToZFB.Req req = new SendMessageToZFB.Req();
req.message = mediaMessage;
req.transaction = buildTransaction("image");
bmp.recycle();
App.getAlipayApi().sendReq(req);
}
private static void sendOnlineImage(String url) {
APImageObject imageObject = new APImageObject();
imageObject.imageUrl = url;
APMediaMessage mediaMessage = new APMediaMessage();
mediaMessage.mediaObject = imageObject;
SendMessageToZFB.Req req = new SendMessageToZFB.Req();
req.message = mediaMessage;
req.transaction = buildTransaction("image");
App.getAlipayApi().sendReq(req);
}
private static boolean isAlipayIgnoreChannel() {
return App.getAlipayApi().getZFBVersionCode() >= 101;
}
private static String buildTransaction(final String type) {
return (type == null) ? String.valueOf(System.currentTimeMillis()) : type + System.currentTimeMillis();
}
}
接收分享返回的信息(比如分享是否成功还是失败了) 创建一个名为apshare的文件夹,在里面创建一个ShareEntryActivity.java(文件名都是固定的,不然接收不到) 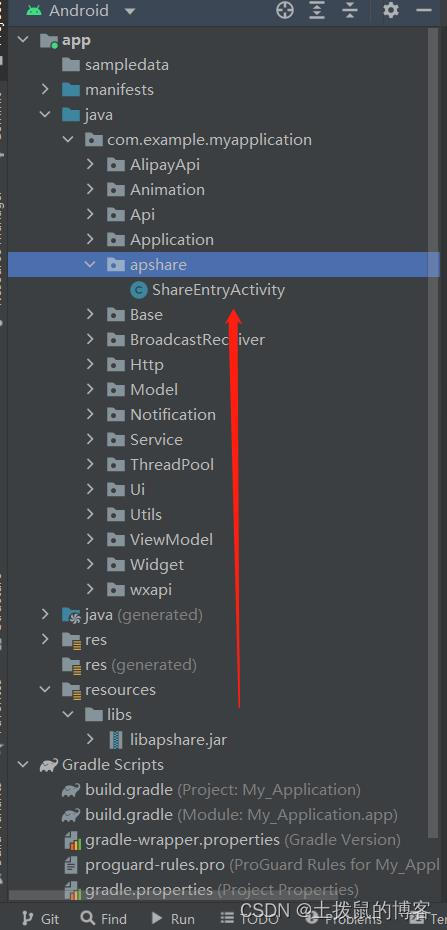 ShareEntryActivity.java 这个ShareEntryActivity其实就是个Activity 页面,只不过没有视图展示出来。 所以调用完毕后必须调用finish();返回上一页。
package com.example.myapplication.apshare;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Intent;
import android.graphics.Color;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.view.WindowManager;
import android.widget.Toast;
import androidx.annotation.RequiresApi;
import com.alipay.share.sdk.openapi.BaseReq;
import com.alipay.share.sdk.openapi.BaseResp;
import com.alipay.share.sdk.openapi.IAPAPIEventHandler;
import com.example.myapplication.Application.App;
public class ShareEntryActivity extends Activity implements IAPAPIEventHandler {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Intent intent = getIntent();
App.getAlipayApi().handleIntent(intent, this);
}
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
setIntent(intent);
App.getAlipayApi().handleIntent(intent, this);
}
@Override
public void onReq(BaseReq baseReq) {
}
@Override
public void onResp(BaseResp baseResp) {
String result = "";
switch (baseResp.errCode) {
case BaseResp.ErrCode.ERR_OK:
result = "分享成功";
break;
case BaseResp.ErrCode.ERR_USER_CANCEL:
result = "错误用户取消";
break;
case BaseResp.ErrCode.ERR_AUTH_DENIED:
result = "错误验证拒绝访问";
break;
case BaseResp.ErrCode.ERR_SENT_FAILED:
result = "错误发送失败";
break;
default:
result = "错误代码未知";
break;
}
Toast.makeText(this, result, Toast.LENGTH_LONG).show();
finish();
}
}
注意这个ShareEntryActivity是要注册到AndroidManifest.xml的,不然也会导致接收不到回调。
- 就跟你注册activity页面一样注册就行了。
- 但是exported必须为true才能接收到其它APP返回的参数。
<activity
android:name=".apshare.ShareEntryActivity"
android:exported="true"
android:theme="@android:style/Theme.Translucent.NoTitleBar"/>
完结~怎么调用就请各位道友自行琢磨了([手动狗头]调用总该会吧),有什么问题可以留言。
|